Page 1 of 3 Finding out how to create a User Interface (UI) using the Java Swing library is not only a useful skill, it also is an ideal way to learn about objects and to make sure that the ideas really have sunk in. So we need to go back to Swing one more time.
A User Interface (or UI) is an essential for a desktop or web application and creating one is very easy using the Swing Library that we first met in Chapter 2. In this chapter we take a closer look at the way you can work with UI objects in both code and within the drag-and-drop designer.
Modern Java With NetBeans And Swing
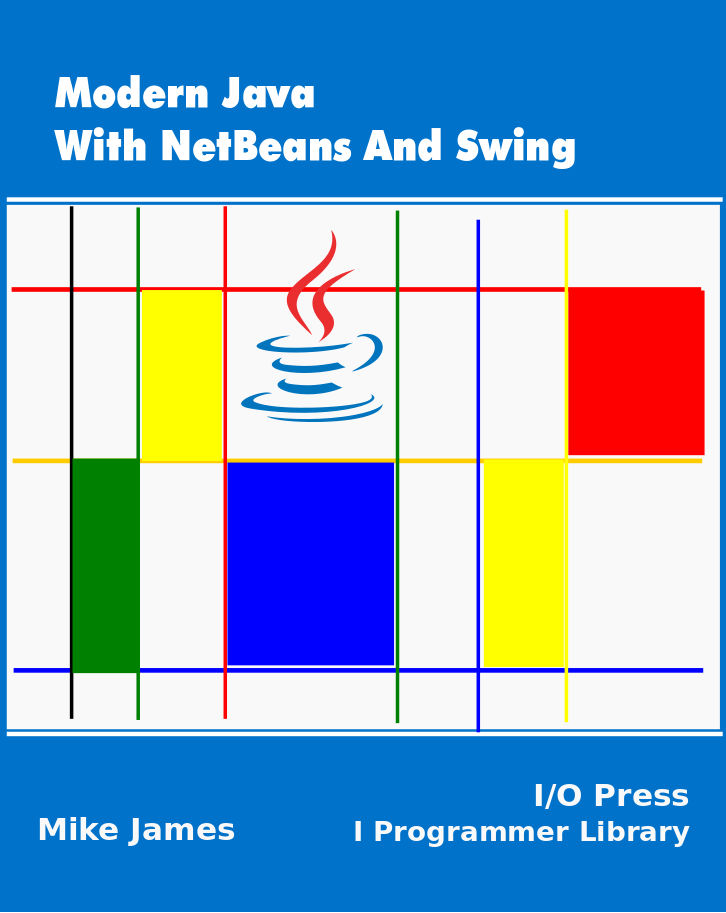
Contents
- Why Java?
- Getting started with Java
- Introducing Java - Swing Objects
- Writing Code
- Command Line Programs
- User Interface - More Swing
- Working With Class
- Java Class Inheritance
- Java Data Types - Numeric Data
- Java Data Types - Arrays And Strings
- Building a Java GUI - Containers
- Advanced OOP - Type, Casting, Packages
- Value And Reference
- Java Lambdas, SAMs And Events
In Chapter 2 we looked at the very basics of Swing in this chapter we go deeper and discover more about the controls that are available to build a user interface. On the way we look at how properties are implemented using get and set methods and started the study of events and how to use them.
A button and some Text
Starting right at the very beginning, the two most basic UI components are buttons and text. It seems natural to think of the button as a UI component but surely text is just text? The fact of the matter is that everything in the UI is built in the same way and any text that you want to display is just as much a UI object or component as anything else.
Let's create a simple example.
Using NetBeans (see Chapter 1), start a new Java Application and call it UITest.
When the project opens right click on the Source Packages folder in the Projects window and select New, JFrame Form.
Call the new JFrame MyJFrame.
As explained in Chapter 2, a JFrame is a basic display window and you can place other UI components within it using the drag-and-drop editor.
To get us started lets drag a button, a jButton, onto the JFrame.
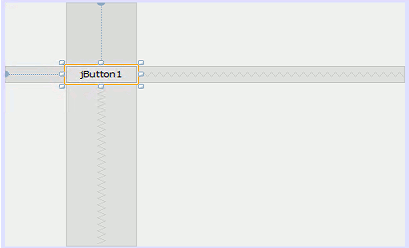
The strange lines and shadings that appear are designed to help you position the button and UI components in general - more of how this works later.
Now if we run the project - click the green button or use Run, Main Project menu option and select MyJFrame as the Main Class - then the code will start being executed - where exactly?
A program generally has a single place to start running and in our case we need to specify that MyJFrame.java is the place to start. If you select Run then you can specify the class where the code starts executing - MyJFrame in this case.
Alternatively you can specify there the code starts using the command Project,Properties and select Run in the dialog box that appears and specify where the program starts.
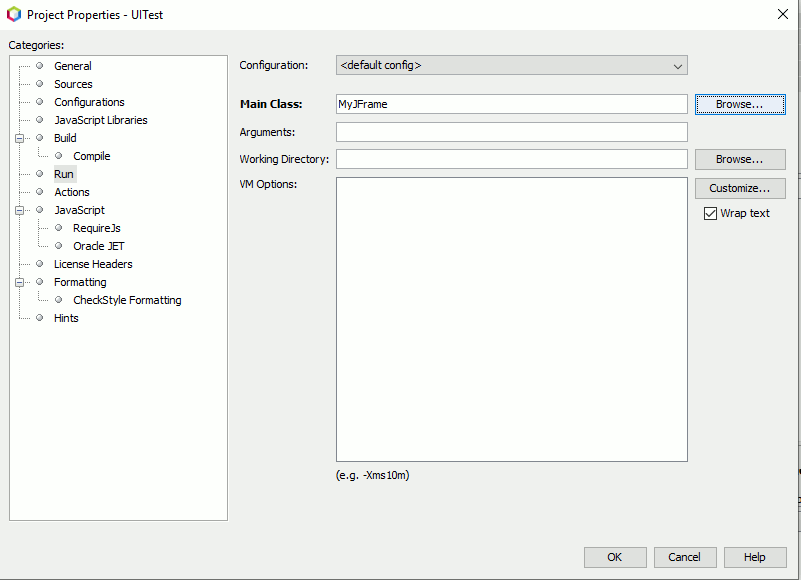
The button that you have placed on the form is an example of a UI object and you can create objects using the drag-and-drop editor in this way or you can write code that does the same job. In Java everything is an object and a jButton is just an example of a jButton object. We will find out soon how to create objects in code but for the moment it is easier to let the drag-and-drop editor do the work - it is usually the easiest way to create a UI. What is important at this stage is that you realize that the editor isn't doing anything magic that is outside of Java. All it is doing is generating the Java code that you would have had no choice but to write yourself before the editor was implemented.
Next drag and drop a Text Field object onto the design surface. Use the mouse to size and position this second object.
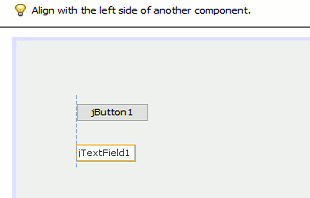
Notice the way the editor gives you helpful hints about how your new component lines up with existing components. It trys to help you layout the overall Ui - but you don't have to take notice of it if you know better.
You could continue to add UI components like this to build up a much richer and more complex form. However for the moment let's concentrate on the button and the Text Field.
Objects, all objects have properties, methods and events that they can respond to.
Let's look at each in turn.
|