Page 1 of 3 The function object is the most important object in all of JavaScript and yet we tend to forget that it is an object. Understanding that JavaScript functions are objects is probably the most important step you can take in understanding the language.
This is an extract from the book Just JavaScript by Ian Elliot.
Buy Now: from your local Amazon
Just JavaScript An Idiomatic Approach
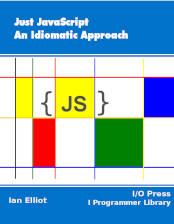
A Radical Look At JavaScript
Most books on JavaScript either compare it to the better known class based languages such as Java or C++ and even go on to show you how to make it look like the one of these.
Just JavaScript is an experiment in telling JavaScript's story "just as it is" without trying to apologise for its lack of class or some other feature. The broad features of the story are very clear but some of the small details may need working out along the way - hence the use of the term "experiment". Read on, but don't assume that you are just reading an account of Java, C++ or C# translated to JavaScript - you need to think about things in a new way.
Just JavaScript is a radical look at the language without apologies.
Contents
- JavaScript – Essentially Different
- In The Beginning Was The Object
- Real World Objects
- The Function Object
Extract - The Function Object Extract - Function Object Self Reference
- The Object Expression
- Function Scope, Lifetime & Closure
Extract Scope, Lifetime & Closure Extract Execution Context ***NEW!
- Parameters, Returns and Destructuring
Extract - Parameters, and Destructuring
- How Functions Become Methods
- Object Construction
Extract: - Object Factories
- The Prototype
Extract - ES2015 Class and Extends
- Inheritance and Type
- The Search For Type
- Property Checking
Buy Now: from your local Amazon
Also by Ian Elliot JavaScript Async: Events, Callbacks, Promises and Async Await Just jQuery: The Core UI Just jQuery: Events, Async & AJAX
<ASIN:1871962579>
<ASIN:1871962560>
<ASIN:1871962501>
<ASIN:1871962528>
In this chapter we meet the most important object of all – the Function object. What really matters is that you don't think that it is just a function.
In JavaScript there are no functions – only Function objects.
As we keep reiterating JavaScript is an object-oriented language, but not in the sense that you might know from languages such as C++, Java or C#, say. JavaScript is different and in the style of Smalltalk and similar dynamic object-oriented languages where everything is an object.
Other object-oriented languages break the "everything is an object" rule, most crucially when it comes to functions, but JavaScript doesn’t.
The mainstream object-oriented languages give in to the very natural pressure to make code special.
You want to write a program – so you want to get on and write some code but where does that code live?
In non-object based languages there is only the code. You start to write a list of statements, and that is all there is to the program.
So when you first start writing programs you tend to think that the code is the most important thing, but later you learn about objects and you are told that code is just a property of an object, i.e. the object's methods.
That is, the language has objects and objects have properties which are usually other objects, and they also have methods which are blocks of executable code.
That is, the answer to the question of where does the code live, in the case of an object-based language, is that code exists as methods which aren't objects but a special type of property that an object can have.
Objects are the main entity in the program and can be assigned and passed around. The code is always bound to some object or other and so you can't do things like pass code as a parameter because it isn't an object. This makes tasks such as event handling and callbacks difficult.
So in most object based languages there are objects and there are methods – which are executable properties.
Constructing Function Objects
JavaScript takes a different approach to the problem of code by introducing the idea of the Function object.
A Function object is created in the way any built-in object is, via a suitable constructor, and used in the way all objects can be used.
To create a new Function object you use:
new Function();
For example:
var myFunction= new Function();
The use of new is optional.
Once created you can add properties to the new object in the usual way:
myFunction.myProperty="hello function";
The point that is being made is that the Function object is "just an object like any other".
So what additional characteristics does the Function object have that makes it useful?
The simple answer is that a Function object can accept some code as part of its creation.
Of course we now need to know what statements JavaScript supports that we can use in the code of a Function object. For the moment only a very simple set of statements are used.
When you create a Function object you can specify a list of statements that form the function’s "body".
For example:
var myFunction= new Function( "alert('hello function body');");
This creates a Function object with a body that consists of the single statement:
alert('hello function body');
So a Function object has some code stored within it.
This code is immutable after the Function object has been created – you can't modify it. If you want to change the code you have to create a new Function object.
The code may be immutable but it is specified as a String and this means that it can be the result of a String expression which computes the code at runtime. It is this possibility that makes it impossible to optimize this way of specifying a Function.
You also need to take careful note that the code that constitutes the body isn't executed when the Function object is created. The body can be thought of as a sort of default property of the Function object – i.e. the Function object simply stores the code for you. In fact there is a [[Call]] property that only Function objects have, but it is an internal property and not accessible within JavaScript code.
All you can do with the function body is execute the code it by using the function invocation operator ().
For example:
myFunction()
causes the list of statements that make up the Function object's body to be executed. In this case it simply causes an alert box to appear with the message.
Notice that:
myFunction;
without the function invocation operator, is a variable that references the Function object and:
myFunction();
i.e. with the function invocation operator, evaluates the body of the Function object that myFunction references.
It is also important to realize that Function objects are anonymous just like all JavaScript objects. That is, they do not have names built-in to their definition. They simply have variables that reference them. This is very different from most other languages and notice that this also applies to function expressions and function statements – see the later section.
In JavaScript, functions do not have names just references.
Function Variables
The code in the function body can declare local variables and these are not properties of any object. Local variables only come into existence when the function is executed and they are destroyed i.e. removed from memory when the function finishes. They are also private to the function and cannot be accessed by any other code. They can be accessed by functions that are defined within the function concerned and this is something we need to consider in more detail later.
To create a local variable you have to use the var keyword:
var myLocalVariable=0;
This creates a local variable which only exists while the function is executing. The unexpected detail is that due to hoisting, see later, local variables exist for the entire lifetime of the function irrespective of where they are declared.
If you don’t use the var keyword then the variable is interpreted as a property of the global object. For example:
myLocalVariable=0;
is the same as:
this.myLocalVariable=0;
As explained earlier, properties of the global object behave like global variables in other languages.
One side effect of using properties rather than global variables is that when you write:
myVariable=0;
then if the property doesn’t exist it will be created. What this means in practice is that forgetting to use var in a function adds properties to the global object that you probably never intended to add. Most IDEs and smart editors will warn you if you don’t use var in a function. In practice it is better to always use this to access or create a property or var to create a variable.
Strict Mode
In strict mode you have to use var in a variable declaration. If you don’t then a runtime exception occurs. Also in strict mode the global object ‘this’ inside a function is undefined. This means a function cannot easily create a global variable or access the global object. Notice that this doesn’t stop a function from using a global variable, only creating a new one.
|