Page 1 of 3 When JavaScript was first introduced to the world it didn't have a dedicated Array data structure - it simply didn't need one, but it got one nevertheless!
Here we look at the Array object, which is easy to use, but can cause a lot of confusion if you don't think about it in the right way.
JavaScript Data Structures

Contents
- The Associative Array
- The String Object
- The Array object
- Speed dating - the art of the JavaScript Date object
- Doing JavaScript Date Calculations
- A Time Interval Object
- Collection Object
- Stacks, Queue & Deque
- The Linked List
- A Lisp-like list
- The Binary Tree
- Bit manipulation
- Typed Arrays I
- Typed Arrays II
- Master JavaScript Regular Expressions
* First Draft
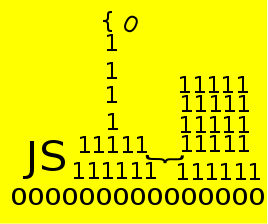
JavaScript is a strange, but perfectly logical, language if you are more familiar with Java, C++ or C#, say. For example, in JavaScript every object is an associative array. In fact it would be fairer to say that the associative array is the fundamental data structure in JavaScript and this is so powerful it can be used to implement object or just about any other data structure you care to think up. Really you don't need anything else.
However, if you do approach JavaScript from the perspective of another language then you will expect to find something that behaves like a traditional array. When JavaScript was first introduced to the world it didn't have a dedicated Array data structure - it didn't need one and in many ways it still doesn't. The associative array is quite sufficient but to make JavaScript easier to use it does have an Array object. This is easy to use but it can cause some confusion if you forget that every data structure in JavaScript is also an object, or more importantly an associative array.
The Array Object
JavaScript provides a special built-in object that behaves much more like a standard indexed array - the Array object - than the more basic associative array.
This inherits from Object so, just like every other object in Javascript, it is also an associative array. However, it is extended to handle arrays with integer indices in a way that corresponds much more to the way indexed arrays work in other languages.
That is, an Array object looks like a standard linear array of the sort that you find in almost any other language - but there are some things that make it behave in ways that you might not expect.
As in the case of most languages, the Array object is indexed by an integer. However, unlike most languages you don't have to specify the size of the Array before you start using it.
For example in JavaScript you can simply create an Array object and start storing values in elements:
var myArray=new Array(); myArray[0]="A"; myArray[1]="B"; myArray[2]="C";
and so on. In this case you can select any element of the array by index e.g. myArray[1] is "B".
You can also create an array object using an Array literal to initialize it. For example:
myArray=["A","B","C"];
creates exactly the same array as the previous example.
Notice that:
myArray=[];
is a common idiom used to create an uninitialized array.
As a third way of creating and initializing an Array object, you an also include the initial values in the constructor function:
var myArray=new Array("A","B","C");
All three methods tend to be used in practice. Which is preferable depends on circumstance, but there are advantages to the array literal notation.
The length of an Array
One of the extras that the JavaScript Array object has is a length property. This keeps track of the size of the array so that you can make use of this information in for loops and the like.
The length property looks a lot like declaring the size of the array, but it isn't quite the same as, say, the dimension specifier in a static language.
The first big difference is that length is updated as you use the array. That is, length is always one more than the largest index you have used.
For example, after:
myArray=[]; myArray[20]="A";
length is set to 21 corresponding to the 21 elements from myArray[0] to myArray[20] . Notice that only element 20 has been initialized and all of the elements from myArray[0] to myArray[19] return undefined if accessed.
You can create an array and set its length using the constructor to specify the final element of the array. For example:
var myArray=new Array(10);
sets the length of the array to 11 i.e. elements 0 to 10. All of the elements are set to undefined.
Notice that this means you can't use the constructor method to create an array with a single element. You also can't write things like:
var myArray=new Array(1.5);
as this doesn't specify the length of an array.
You can also set the length of an array after it has been created by assigning directly to the length property. For example:
myArray=[]; myArray.length=20;
sets the length of the array to 20 with all of the elements still uninitialized.
If you set the size of an array to be larger than it currently is then not much actually happens. However, if you set the size of an array to be smaller than it currently is, then you lose elements. For example:
var myArray=new Array("A","B","C"); myArray.length=1;
alert(myArray[1]);
displays undefined because after setting the array's length to 1 only myArray[0] is left.
You can see that an Array behaves a lot like a standard JavaScript object but with the addition of a length property that keeps track of the highest integer key you have used. This is indeed more or less what an Array is.
Working with Array
Notice that you can refer to array elements that don't currently exist. That is, unlike in other languages, you don't have to "declare" the length of an array before you use it.
If you attempt to access an element that hasn't been initialized then undefined is returned. For example:
myArray=[]; alert(myArray[50]);
displays "undefined".
If you assign to an element that doesn't currently exist then that element is created. For example:
myArray[50]=10; alert(myArray[50]);
displays 10, but if you try to display myArray[40] you will discover that its value is still undefined.
Put simply:
- When you create an array object you don't have to specify its length - elements are created as you store values in them.
- Any elements you access that you haven't stored a value in return undefined.
- The array keeps a record of "how long it is" in its length property.
- The length of an array is simply one more than the highest indexed element you have used.
- You can set the length property by direct assignment or via the Array constructor.
Iterating The Array
One of the reasons that we often use an Array is that we need to process each one of the elements in turn.
There is a natural pairing between the Array and the standard for loop in every language.
For example:
for(i=0;i<myArray.length;i++){ alert(myArray[i]); }
That is i goes from zero, the first array element to the length of the Array minus one i.e. the last array element.
You could say that the array was invented to give the for loop something to do or perhaps it was the for loop that was invented to make working through Arrays easier.
Notice that in JavaScript there is the possibility that you will encounter Array elements that haven't been initialized, i.e. they will be undefined.
It is also better to avoid using the for in loop with an Array because this iterates over all of the properties of the Array object, and not just the elements of the Array. It is even more complicated than this because properties that are set to DontEnum are left out of the iteration. In short, if you use for in you can't be 100% sure that you are going to get just the elements of the array.
There is also another big difference that can occasionally be useful is that for in only iterates over the array elements that are defined. For example:
var myArray=new Array("A","B","C"); myArray[5]="E"; for (x in myArray) { alert(myArray[x]); }
In this case a for loop would display six elements with myArray[4] displaying as undefined. The for in loop, however, skips the undefined element. This might seem like a neat way of doing things but it probably isn't worth the risk of it not working due to other properties being included in the loop. There is also the small problem of the index variable, x in this case, being a string rather than an integer.
In general, if you need to iterate through an Array, use a standard numeric for loop. It is both safe and fast and it fits into the "ethos" of using an Array so much better. Save the for in loop for data structures that aren't indexed by integers.
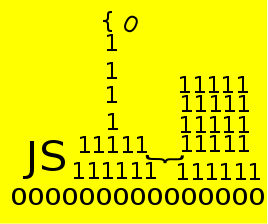
|