Page 1 of 4 JavaScript is a very subtle and sophisticated language and it deserves to be treated in its own right and not as a poor copy of other object-oriented language. In this first chapter of Just JavaScript we take a radical look at the way everything in JavaScript is an object.
Just JavaScript
There is a newer version of the draft of the book here.
A Radical Look At JavaScript
Contents
- JavaScript Isn't Java, or C, or C# ... (Book Only)
- In The Beginning Was The Object
- The Function Object
- How Functions Become Methods
- The Object Expression
- Object Construction
- The Prototype
- Type And Non-Type
- Constructor And InstanceOf
- Duck Testing And Prototype Construction
-Preface-
Most books on JavaScript either compare it to the better known class based languages such as Java or C++ and even go on to show you how to make it look like the one of these.
Just JavaScript is an experiment in telling JavaScript's story "just as it is" without trying to apologise for its lack of class or some other feature. The broad features of the story are very clear but some of the small details may need working out along the way - hence the use of the term "experiment". Read on, but don't assume that you are just reading an account of Java, C++ or C# translated to JavaScript - you need to think about things in a new way.
Just JavaScript is a radical look at the language without apologies.
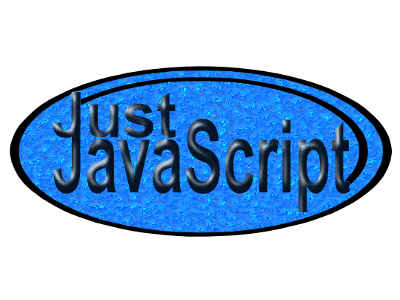
-Introduction-
The trouble with JavaScript is that it isn't like the other mainstream languages that are in common use. It may have Java in its name but that's all it shares with this class-based, object-oriented language.
JavaScript isn't Java or any class based object oriented language re-written, it is something very different.
The Script part of its name is equally misleading. Yes, it can be used to add a few lines to make a web page do something and in this sense can be used as a casual scripting language, but it is also a fully fledged language of surprising power.
What all this means is that if you are using JavaScript correctly and appropriately it should look nothing like Java and nothing like Script.
What is more when you get to know it you discover that it is a minimalistic sort of language that lets you do some very advanced things. In fact JavaScript resembles Lisp in the way that it can be shaped to suit the programmers tastes and ambitions. This is a good thing but it is also a curse because programmers spend a lot of time turning JavaScript into somthing they recognise rather than recognising what is different about JavaScript.
You need to take JavaScript seriously.
What about all the terrible things you may have heard about the language - are they true?
Some are, as JavaScript is far from perfect. However, many of the criticisms are the result of misunderstanding how the language works and what it is trying to do.
So to get the best from JavaScript you need a thoroughly modern introduction that isn't just a rewrite of something aimed at Java or C++ or any class-based language. You need an introduction that presents JavaScript as a language with its own philosophy and approach and this is, I hope, what you are reading.
In what follows it is assumed that you already have some idea of how to program. Nothing deep or advanced, but you should know what a for loop is and what an if statement does. These ideas will be introduced, but not with the gentle pace that a 100% complete beginner needs.
In the beginning was the object { }
JavaScript is not just an object-oriented language it is object-based. That is, in JavaScript the object is the fundamental entity.
In other languages, as well as objects, we have classes and types and these are in many ways more fundamental than the objects. JavaScript doesn't have classes and it doesn't have types, but it can be made to appear to have both if you insist - it is a language powerful enough to mimic the way other languages work.
In JavaScript everything is an object
(with the exception of null and undefined which we can ignore until later).
When you work with JavaScript you generally start by creating objects that you want to use.
An object is a container that can contain a collection of other objects.
The starting point for all of this is the empty object literal:
{}
The curly brackets mean that this is an object and it can contain other objects within the curly brackets. Believe it or not this is a valid JavaScript program. If you run it, it creates an empty object which promptly disappears again as the program comes to an end.
So what can you put inside an object?
The simple answer is that an object can store a list of name object pairs written as
name:object
with a separating colon.
These are generally known as the properties of the object
Multiple properties are entered as a comma separated list.
For example:
{prop1:object1,prop2:object2, and so on}
At the moment we only know about the empty object so it is difficult to give an example that doesn't look either silly or amazingly abstract depending on your point of view. But as the idea has been raised here is a simple non-null object:
{object1: {}, object2: {}, object3: {}}
This is an object with three properties called object1, object2 and object3. Each of these properties is a null object and hence the whole thing isnt very useful.
However, it is worth pointing out the you can already build objects that are nested and hence tree like.
For example:
{object1: {}, object2: {object3: {} } }
This has object1 and object2 at the "top level" and object3 nested within object2. You can see that this is completely general and makes JavaScript objects tree-like structures.
There is a second and more standard way of creating an empty object:
new Object();
has the same effect (almost) as using {} i.e. it creates a new empty object. The use of {} is a syntactic change designed to make object creation easier.
Property Access
Now we come to how to access the properties of an object.
There are two equivalent ways of doing this. The first is to use the dot notation. If you want to specify a particular property of an object you simply write:
object.property
The second is to use square brackets and write:
object[property]
Both expressions means the same thing and they give you the object associated with the property.
Which notation you use depends on a number of different things, but in most cases you should use the dot notation unless you have a good reason not to.
Later on you will discover that the form:
object[property]
has one advantage that is sometimes vital. The property can be specified by a string which means you can access a property dynamically, i.e. the property accessed can only be determined at run time. You can think of this form of property access as being more like accessing an array of values. The dot form of the property is fully determined at "compile" time and this can allow some optimisations.
If the property is another object and this has properties then you can simply use the same principle again.
For example:
object.property1.property2
and so on
or:
object[property1][property2]
and so on.
Referring to a property in this way can be used to change its value. For example:
object.property1={}
sets property1 to a new empty object.
You can also create a new property, i.e. add a property to an existing object, by simply assigning something to it.
For example:
object.property3={}
If the property exists then it is set to a new empty object, but if it doesn't currently exist it is created and initialized to a new empty object.
it is the ability to assign values to properties that don't currently exist that makes JavaScript objects interesting. They can be dynamically extended by assignment and this means
all JavaScript objects are dynamic.
|