Page 1 of 3 Now that we know all about how to get a file, it is time to post some data back to the server. In this chapter we look at both get and post as ways of sending data and see how to send form data under Ajax control.
Just jQuery Events, Async & AJAX
Is now available as a print book: Amazon
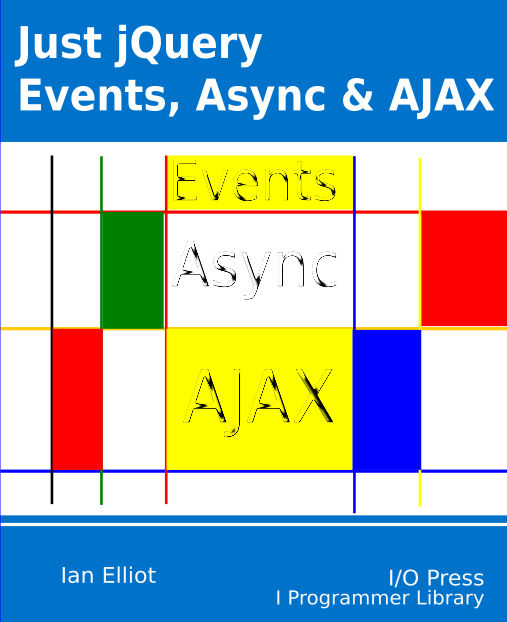
Contents
- Events, Async & Ajax (Book Only)
- Reinventing Events
- Working With Events
- Asynchronous Code
- Consuming Promises
- Using Promises
- WebWorkers
- Ajax the Basics - get
- Ajax the Basics - post
- Ajax - Advanced Ajax To The Server
- Ajax - Advanced Ajax To The Client
- Ajax - Advanced Ajax Transports And JSONP
- Ajax - Advanced Ajax The jsXHR Object
- Ajax - Advanced Ajax Character Coding And Encoding
Also Available:
buy from Amazon
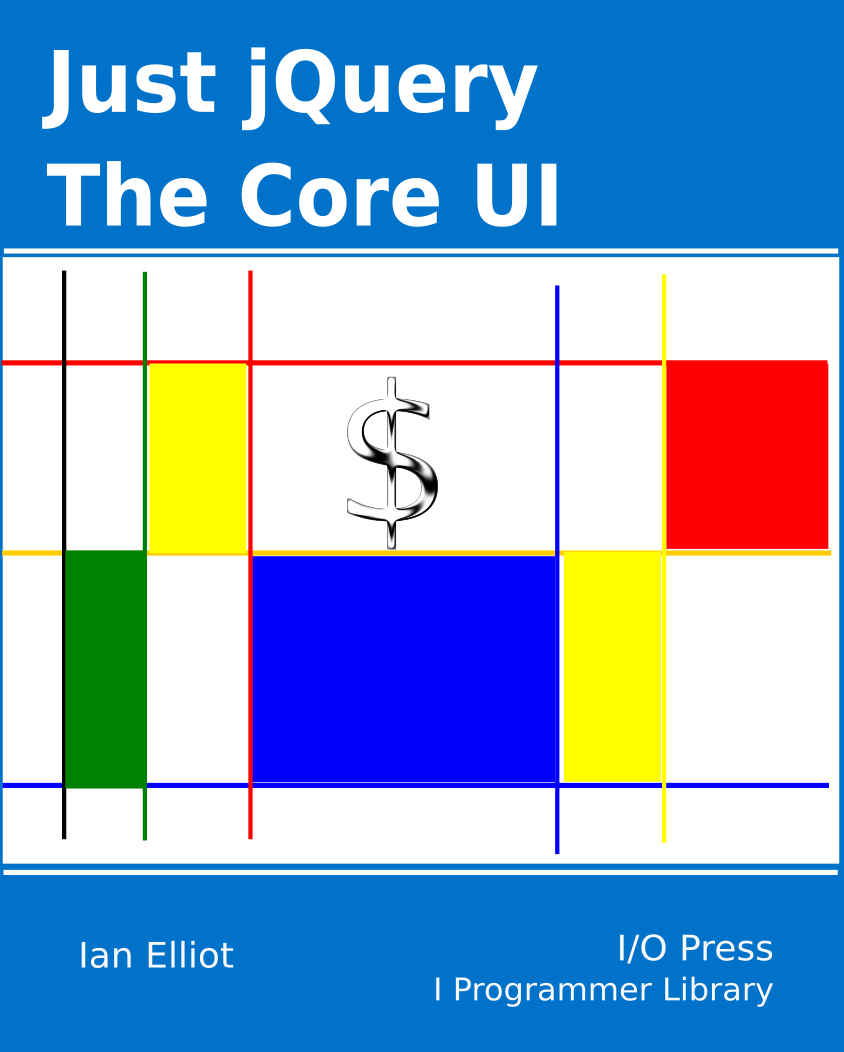
Advanced Attributes
As explained in the previous chapter, both get and post retrieve data and both can send data to the server we tend to use get to retrieve data and post to send data. Post was designed to send data to the server as suggested by its name but it proved important to have a way to send some data to the server even when using get and to achieve this we started to use the URL itself as a data transport.
Let's take a look at the simplest uses of post.
Post
The jQuery post command is very easy to use but it can be difficult to get everything right on the client and on the server.
The basic command is works exactly like get:
$.post(url)
which retrieves the file specified by the url and returns a jqXHR Promise object. You can specify a success callback as a parameter but as in the case of get it is easier to use the Promise object to define any callback.
For example:
$.post("myData.txt").then( function(data){ alert(data) });
this simply asks the server for the file myData.txt stored in the same directory as the page making the request.
You can use the then, catch and always Promise methods as described in the previous chapter.
This all works but it is unusual to simply use a post request to retrieve the contents of a file. A post usually sends data to the server in the body of the request and jQuery lets you specify this data a the second parameter.
In principle you can send the data in any format you like as long as the server is able to deal with it. In practice jQuery provides automatic processing for a number of standard formats and it is easier to stick with these.
For example the simplest format to use is to send key value pairs which jQuery will extract from an object you supply. So for example if you want to send a first name, second name and an id number you could use:
var sendData={first:"ian",second:"elliot",id:27}; $.post("myData.txt",sendData).done( function(data){ alert(data) });
This sends the data to the server but of course the server just ignores it and sends the requested page back to the client.
To make use of the data there has to be a custom handler on the server to process the data. There are so many ways of doing this ranging from traditional CGI, JavaServlets, Node.js and so on.
For the sake of a concrete example we will use PHP to provide the server side processing.
The key idea is that the page that the post requests is best regarded as a program that processes the data and optionally returns a result to the client.
As long as you send data to a PHP page in key value pairs PHP will do you the favour of decoding them and will make them available as an associative array $_POST. This means that you have virtually no extra work to do on the server side to extract the data from a post.
You can also check that the request sent to the server was a post by testing the $_POST variable:
if($_POST){process data};
You also need to know that dots and spaces in the key will be converted to underscores e.g. a key like "I Programmer.info" is automatically converted to "I_Programmer_info".
You can handle the data sent by the previous example using a PHP file process.php something like;
<html> <head> <meta charset="UTF-8"> <title></title> </head> <body> <?php echo( $_POST['first']); ?> </body> </html>
which sends the first name back to the client within a web page.
PHP also automatically processes the value that you pass into either a string or an array according to its format. Notice that how and how much the data is preprocessed is a function of PHP not of the post request or Ajax transactions in general.
The general idea is that what ever method you use to process the data on the server it will usually provide you will some additional help and it is up to you to discover what it is and how to access the data.
So for example if we post the data:
var sendData={first:"ian", second:"elliot", id:27.5, array:[1,2,3]};
It could be processed in a PHP file something like:
echo(gettype($_POST["array"][1]));
which would return the string "2".
Notice that the array is a string array even though the values are numbers. If you want to work with numeric values then it is up to you to convert the data. This is because everything sent in an HTTP packet is text - there are no data types in the interaction.
Once again it is worth mentioning that it is the server side system you use that provides the data processing facilities and hence these are going to vary according to what you use.
If you are using PHP then it is worth looking up the filter_input function which can perform validation and conversion as well as sanitization of input. For example if you want the id field to be returned as an integer you could use:
$id=filter_input(INPUT_POST,"id", FILTER_VALIDATE_INT);
This is by far the best way to work with any input data - post, get, cookie or server. This provision of functions that do the sort of tasks that you actually want to do is one of the reasons PHP is a good choice of server side languages. It may not be elegant but it is very practical.
So to summarize:
- Post sends data in the body of the request and retrieves data from the server but the file specified from the URL is expected to process the data sent.
- The data sent to the server can have any format but there are formats that are better supported than others in particular a string of key value pairs.
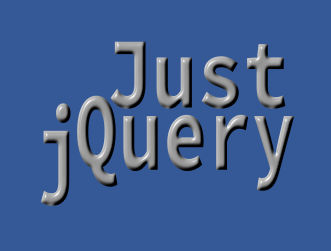
|