Page 1 of 5 So far in our exploration of jQuery we have used the "shorthand" Ajax methods. These are all implemented as calls the to the full jQuery ajax method and if you want to do anything slightly out of the ordinary then you need to make use of it directly. In this installment we look at controlling the request and sending data to the server
Just jQuery Events, Async & AJAX
Is now available as a print book: Amazon
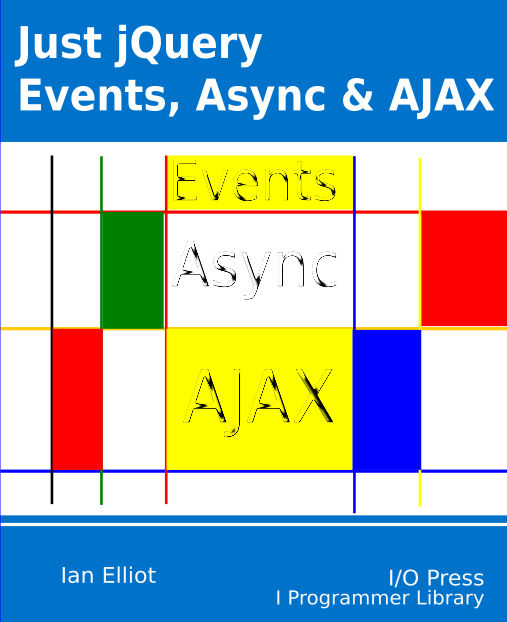
Contents
- Events, Async & Ajax (Book Only)
- Reinventing Events
- Working With Events
- Asynchronous Code
- Consuming Promises
- Using Promises
- WebWorkers
- Ajax the Basics - get
- Ajax the Basics - post
- Ajax - Advanced Ajax To The Server
- Ajax - Advanced Ajax To The Client
- Ajax - Advanced Ajax Transports And JSONP
- Ajax - Advanced Ajax The jsXHR Object
- Ajax - Advanced Ajax Character Coding And Encoding
Also Available:
buy from Amazon
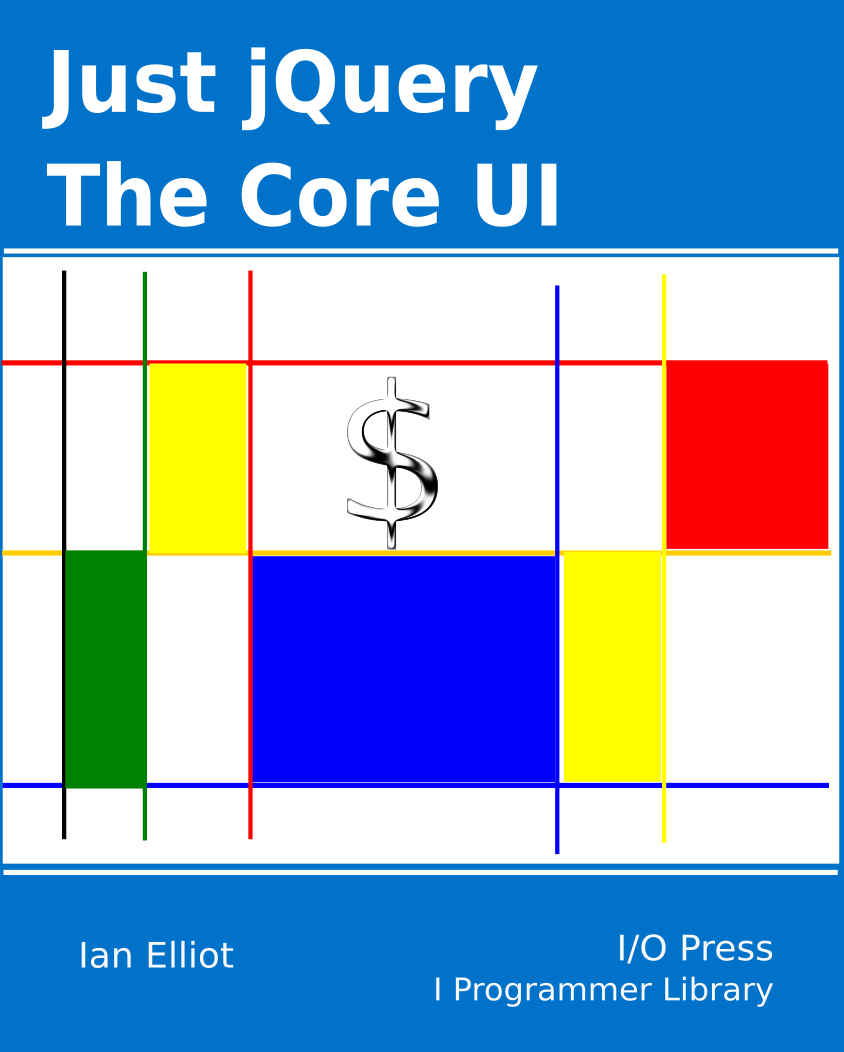
Advanced Attributes
Raw ajax method
The raw or low level ajax method is very easy to specify. You can call it either using:
$.ajax(url,options);
or just as
$.ajax(options);
with the url specified as part of the options object.
All of the complexities of using it are hidden in the options object. This is a set of key value pairs that you use to specify how the Ajax request should be performed.
Notice that the only difference between the two forms of using the ajax method is that if you don't specify the url as the first parameter it has to be specified in the option object.
The simplest post request using the ajax method is something like:
var options={}; options.url="process.php"; options.method="post"; options.data={first:"ian",last:"elliot",id:27};
$.ajax(options).then( function(data){ alert(data); });
That is the url property sets the request url, the method sets the method to post in this case and the data property is the data sent to the server.
This is all there is the the ajax method and all that remains to do is investigate the various options you can set.
Instead of dealing with the option in alphabetical order, which is what the documentation does, it is more useful to look at them in groups according to what they are concerned with.
Controlling the request
There are a set of options that modify the very basic nature of the Ajax request. Instead of just presenting them as an alphabetic list it is worth dealing with the in logical groups.
You need to read the first section on controlling the basic HTTP call.
Basic HTTP call
There are a set of options that control the basic nature of the HTTP request being made and how it is handled.
It is important that you read the section on ifModified and cache.
- url
sets the url of the request. For example: options.url="process.php";
- method or type
method sets the type of request, and you can use type as a alias for method. You can set it to GET, POST. PUT or HEAD. Its default is GET. For example: options.method="post";
- async
you can force a request to be handled in a synchronous way. If you do this then your JavaScript will wait for the request to complete and the UI will freeze. The default setting is true and this is how you generally should leave it. There are very few, if any, uses of synchronous Ajax requests and jsonp in particular doesn't support it.
- ifModified and cache
Setting ifModified to true will cause the Ajax request to fail if the browser detects that the resource hasn't changed since it was last requested. Setting cache to false forces Get and Put to retrieve the resource even if it is available in the cache. ifModified and cache control whether a resource is retrieved over the network or not and this is a topic which deserves separate treatment.
-
timeout
Sets the timeout for the request in milliseconds. The timing starts from the attempt to make the request so it can include time spent queuing for the client to deal with it. What this means is that you might have to factor in additional time over and above the response time of the server.
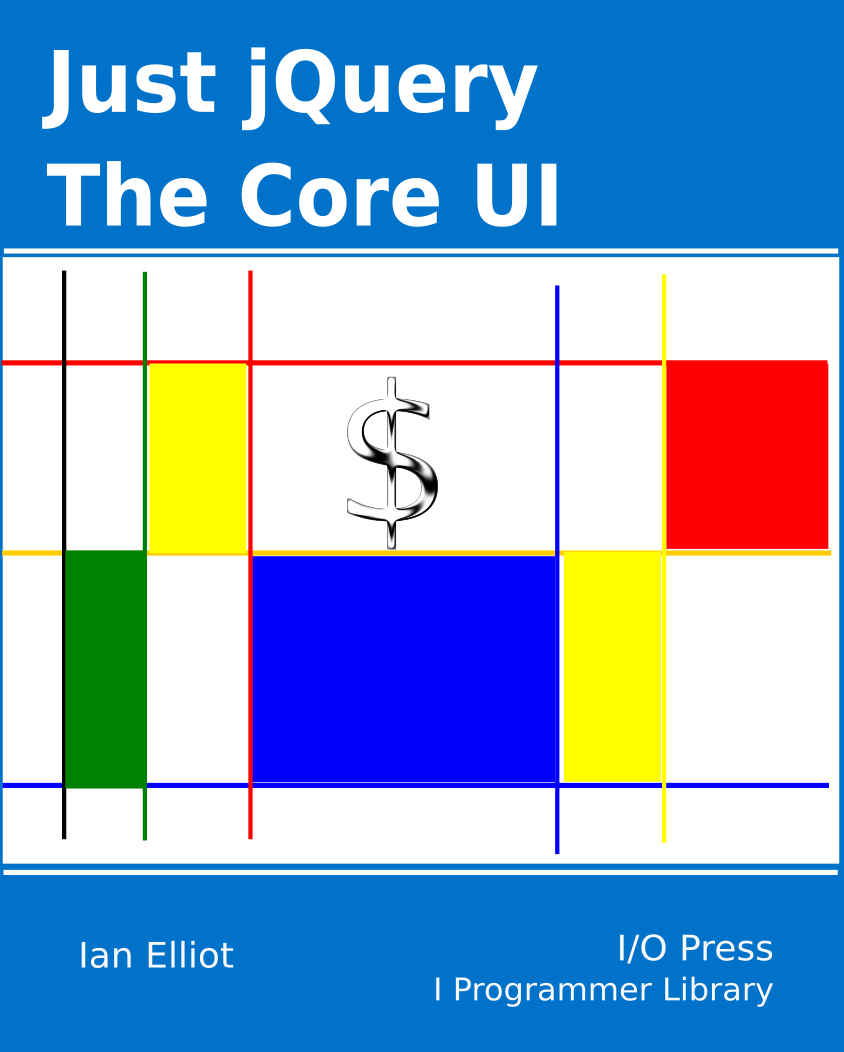
|