Page 1 of 2 Python is object-oriented. In Python everything is an object, but unless you look carefully you might never see one.In this extract from Mike James' forthcoming book, we take a completely different look at objects in Python.
Programmer's Python Everything is an Object Second Edition
Is now available as a print book: Amazon
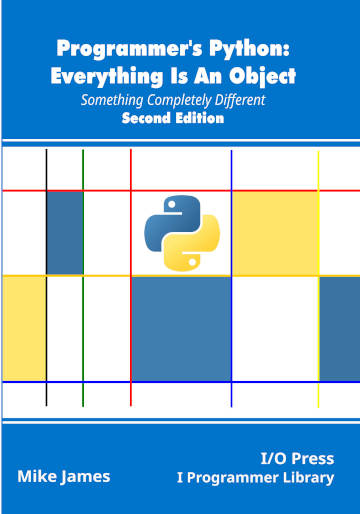
Contents
- Get Ready For The Python Difference
- Variables, Objects and Attributes
- The Function Object
- Scope, Lifetime and Closure
Extract 1: Local and Global ***NEW!
- Advanced Functions
- Decorators
- Class, Methods and Constructors
Extract 1: Objects Become Classes
- Inside Class
- Meeting Metaclasses
- Advanced Attributes
- Custom Attribute Access
- Single Inheritance
- Multiple Inheritance
- Class and Type
- Type Annotation
- Operator Overloading
- Python In Visual Studio Code
Extracts from the first edition
<ASIN:1871962749>
<ASIN:1871962595>
<ASIN:1871962765>
Python is an object-oriented language, but is isn’t a classical class-based, strongly-typed language.
You can learn and use Python for many years without ever knowing anything about its object-oriented features. The reason for this is that Python presents a conventional face to the world in the form of functions. You can write Python functions and use them to build relatively large programs without ever realizing that a Python function is an object.
If you want to know about Python objects then just take a look at any of the many introductions. The problem you will encounter is that most introductions tell you about Python’s object-oriented features by comparison to classical class-based, strongly-typed language and this isn’t what Python is. You can get by understanding Python in this way and you can cope with its differences as a list of “Python doesn’t do”. This works, but it leaves a sense of mystery and magic. It is so much better to understand what the principles are that Python is based on. Then you don’t have to remember seemingly arbitrary rules and exceptions because it all makes sense; it is all obvious.
After the high speed overview of Python in Chapter 1, this chapter lays some of the foundations of understanding Python.
In particular, we take a detailed look at how variables and objects work.
Variables and Objects
The two fundamental entities in Python, and in some form in all languages, are variables and objects. Variables are basically about allowing the programmer to work with objects and there are a number of possible ways of implementing this.
In Python, variables are references to objects and any variable can reference any object.
A variable is created by simply using it.
That is, variables in Python are dynamic, untyped and are references to objects.
If you have a background with a more traditional language this will seem barbaric and an opportunity to create a huge mess.
Objects in Python are simply named collections of attributes. Each attribute is a reference to another object. This is a very simple, but very powerful, data structure.
There is a tendency to think of variables as being things that store objects. This isn’t a helpful way to think about things. You should think of a variable as being a label, pointer or reference to an object which lives elsewhere in memory.

The key idea is that objects and variables have their own existence.
For example, multiple variables can reference the same object.
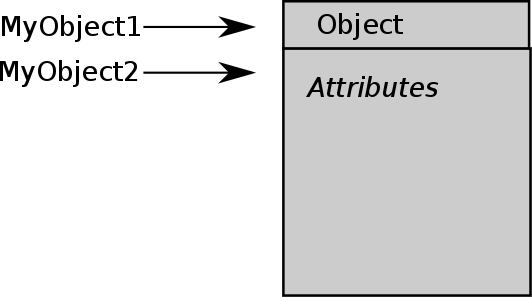
What this also implies is that a variable doesn’t name an object and there is a sense in which all Python objects are anonymous.
Before we can investigate further, we need an example Python object to work with. The simplest way of getting a custom object is to use the class definition. Python, initially at least, only has built-in objects. It also has no direct way of creating an object literal, but the class definition can do the same job.
For example:
class MyObject: myAttribute=1
creates an object called MyObject that has the attribute myAttribute. You can create additional attributes by listing additional variables.
Notice that in other languages what Python calls an attribute would be called a property. Python reserves the term "property" for an attribute with a getter and a setter – see later.
Also notice that the class definition creates a class object, which is generally used for a purpose other than just creating a custom object. It also has a number of predefined properties that, for the moment, we can ignore.
Once you have an object you can access its attributes using the familiar dot notation.
For example:
print(MyObject.myAttribute)
MyObject.myAttribute=2
print(MyObject.myAttribute)
prints 1 followed by 2.
An attribute behaves just like a variable in that it can reference any object without having to be declared as a specific type.
Attributes are untyped.
Now we have an example custom object, it is possible to demonstrate the idea that variables store references to objects.
Despite the fact that the class definition:
class MyObject:
is an object with a name, i.e. MyObject, this isn’t what is happening. You should think of the class declaration as being the equivalent of:
MyObject=class:
where class is something that returns a custom object. MyObject is just another variable and you can assign to it, so destroying the association of the name MyObject with the custom object just created.
That is, after:
MyObject="ABCD"
print(MyObject.myAttribute)
you will see the error
AttributeError: 'str' object has no attribute 'myAttribute'
The variable MyObject no longer references the custom object, but a string object which doesn’t have a myAttribute attribute.
You can also assign the reference to another variable:
MyObject2=MyObject
print(MyObject2.myAttribute)
The point here is that the variable that you might think is permanently associated with the object isn’t and this is the sense in which Python objects are nameless.
Advanced Note: There is a standard attribute of class objects, __name__, which stores the name of the variable that was used in the class definition and in this limited sense Python objects aren’t anonymous.
It is very important that you always think of objects as having an existence all of their own, independent from any variables that might be used to reference them.
This idea becomes really important when we look at function objects in the next chapter.
An object certainly has a lifetime that is independent of the life of any one variable that might reference it. However, if no variables reference an object then it is inaccessible from the program and the Python system will garbage collect it, i.e. remove it from memory and free the resources it once used.
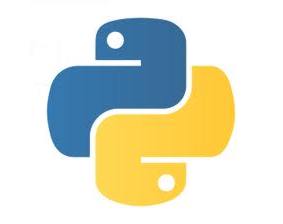
|