Page 1 of 4 So you know how to create an Android app, but do you really know how it works? In this second part of our ebook on getting started with Android using Android Studio 2.3 we look at how to create a UI and how to hook it up to the code in the Activity.
 Android Programming In Java: Starting With an App Third Edition
Is now available in paperback and ebook.
Available from Amazon.
- Getting Started With Android Studio 3
- The Activity And The UI
- Building The UI and a Calculator App
- Android Events
Extract: Using Lambdas
- Basic Controls
- Layout Containers
- The ConstraintLayout
Extract: Guidelines and Barriers
- UI Graphics A Deep Dive
Extract: Programming the UI ***NEW
- Menus & The Action Bar
- Menus, Context & Popup
- Resources
- Beginning Bitmap Graphics
Extract: Simple Animation
- Staying Alive! Lifecycle & State
- Spinners
- Pickers
- ListView And Adapters
If you are interested in creating custom template also see:
Custom Projects In Android Studio
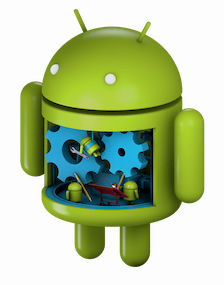
There is an earlier version of this chapter that deals with Android Studio 2.2 and earlier. Android Adventures - Activity And UI With Android Studio 2
We discovered in Chapter 1 how to use Android Studio Google's Android IDE, to build the simplest possible app.
On the way we discovered that an Android app consists of two parts - an Activity and a View.
The Activity is the Java code that does something and the View provides the user interface (UI).
You can think of this duality as being similar to the HTML page and the JavaScript that runs to make it do something, or as a XAML form and the code behind.
The key idea is that an Activity is the code that works with a UI screen defined by the View. This isn't quite accurate in that an Activity can change its view so that one chunk of Java code can support a number of different views. However, there are advantages to using one Activity per view because, for example, this how the Android back button navigates your app - from Activity to Activity.
A complex app nearly always consists of multiple Activities that the user can move between like web pages but a simple app can manage quite well with just one Activity.
There is no hard and fast rule as to how many Activities your app has to have - but it has to have least one.
If you are wondering if an Activity can exist without a View the answer is that it can, but it doesn't make much sense as this would leave the user with no way to interact with your app.
Activities are active when their View is presented to the user.
It really is a great simplification to think in terms of an Activity corresponding to a single screen with a user interface.
If you want something to run without a UI then what you want is a service or a content provider - more of which in a follow on book
It is also worth making clear at this early stage that an Activity has only one thread - the UI thread - and you need to be careful not to perform any long running task because this would block the UI and make your app seem to freeze. That is an Activity can only do one thing at a time an this includes interacting with the user. If you write a program using a single activity and it does a complicated calculation when the user clicks a button then the activity will not be able to respond to any additional clicks or anything that happens in the UI until it finishes the calculation.
In most cases the whole purpose of the Activity that it associated with the UI is to look after the UI and this is what this book is mostly about.
Also notice that creating additional Activities doesn't create new threads. Only one Activity is active at any given time - more of this later when we consider the Activity lifecycle in detail.
In other words, Activities are not a substitute for knowing about threading but if you are just getting started you can ignore these issues for a while.
In this book we are going to concentrate on the single screen UI Activity because it is the most common app building block you will encounter and it is even where most complex apps start from.
The MainActivity
There is of course one activity that is nominated as the one to be launched when you app starts.
If you use Android Studio to create a new Basic Activity app called SimpleButton and accept all the defaults then the startup Activity is called MainActivity by default.
You can change which Activity starts the app by changing a line in the app's manifest.
The Manifest is a project file we haven't discussed before because if you are using Android Studio you can mostly ignore it and allow Android Studio to construct and maintain it for you - but it is better if you know it exists and what it does.
The Manifest is stored in the app/manifests directory and it is called AndroidManifest.xml.
It is an XML file that tells the Android system everything it needs to know about your app - including what permission it requres to run on a device.
In particular it lists all of the activities and which one is the one to use to start the applications.
If you open the generated Manifest, just double click on it, you will see a little way down the file:
<activity android:name=".MainActivity" android:label="SimpleButton" >
This defines the Activity the system has created for you and the lines just below this:
<intent-filter> <action android:name= "android.intent.action.MAIN" /> <category android:name= "android.intent.category.LAUNCHER" /> </intent-filter> </activity>
Define it as the startup Activity.
Notice which Activity starts the app has nothing to do with what you call it i.e. calling it MainActivity isn't enough.
For the moment you can rely on Android Studio to look after the Manifest for you. In most cases you will only need to edit it directly when you need to correct an error or add something advanced.
Inside the Activity
The generated Activity has one class MainActivity which holds all of the methods and properties of your activity.
It also have three generated methods -
- onCreate
- onCreateOptionsMenu
- onOptionsItemSelected
The last two are obviously connected to the working of the OptionsMenu and this is an important topic but one that can be ignored for the moment. Not all Activities need to have an OptionsMenu and you could even delete them if you don't want to support an options menu.
The most important method defined is onCreate.
This is an event handler and it is called when your app is created.
This is the place we do all the initializations and setting up for the entire app. It is also generally the place we show the app's main UI screen.
Let's take another look at the first two lines of generated code for onCreate which are the most important:
protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
The onCreate event handler is passed a Bundle object called savedInstanceState. This is intended to preserve state information between invocations of you app and we will see how this is used later. In this case no data has been saved and so savedInstanceState is null - but you still have to pass it on to the inherited onCreate method.
You will learn a lot more about Bundle later.
The final instruction calls setContentView which is a method that has a number of different overloaded forms.
In this case we pass an integer that indicates which XML file describes the layout to be used for the view. The setContentView method uses this to create all of the components of your UI as defined in the XML file. That is this is the connection between the layout you created using the designer and the layout that appears on the device's screen.
It is worth looking a little closer at the way that the layout file is specified because this is a general way that Android lets you access resources. There is a whole chapter about resources later but it is still worth an introduction now.
The R object is constructed by the system to reflect the resources you have placed in the resource directories. In this case R.layout.activity_main returns an integer value that allows the setContentView method to find the activity_main XML layout file.
In general all resources are found via the R object, think of it as a library of resources.
View and ViewGroup
Ok so far so good but it is important to realize that what happens next it that the XML file is rendered as a set of View objects. That is Java objects that are all sub-classes of the View object.
The entire UI and graphics system is implemented as a hierarchy of components derived from the View class. If you have used almost any GUI framework - AWT, Swing, XAML etc - then this idea will not be new to you.
For example, a button is a class derived from view and to create a button all you have to do is create an instance of the button class. You can, of course create as many buttons as you like simply by creating more instances.
This leaves open the question of where the button appears in the layout?
The answer to this is that there are ViewGroup objects which act as containers for other view objects. You can set the position of the child view objects or just allow them to be controlled by various layout rules - more of which later.
You can opt to create the entire UI in code by creating and working with instances of view objects and this is something demonstrated in a later chapter UI Graphics A Deep Dive.
So to be 100% clear all of the UI objects are defined in code and every UI object like a button say has a Java class with a similar name that lets you create the UI in code.
In fact this is the only way to create the UI but there are other ways of specifying it.
Instead of writing code to create the UI you can specify what you want in an XML file and then use supplied code to display it. This is what setContentView does - it reads the XML file you give it and creates Java objects that implement the UI.
This means you could create the UI by manually writing an XML file that defines view objects and how they nest one within another and rely on the system to create the view object hierarchy for you.
This is possible but it is much easier to use the designer to create the XML file and then allow the system to create the objects for you from the generated XML file. That is you can drag-and-drop a button onto the designer and it will automatically generate the XML needed to create it and specify where it is and all of the other details you set.
That is at the surface level there are three ways to create the UI.
- You can write Java code to generate the necessary Java objects.
- You can write XML tags and use the system to convert the XML to the same Java objects.
- You can use the designer to interactively create the UI and generate the XML file which is then converted into the Java objects needed.
You can think of this as:
drag-and-drop layout -> XML -> Java View objects
Being able to work with the UI in an interactive editor is one of the great advantages of using Android Studio and even if you know how to edit the XML layout file directly it isn't a feature you should give up lightly. It is nearly always a good idea to use the layout editor at first and apply any tweaks, if necessary to the XML file later.
|