Page 1 of 4 So far we have overlooked resources, but the subject can be ignored no longer. Resources serve too important a purpose in Android. They not only make localization easier, they are key to creating Resourcesapps that adapt to the device they are being run on.
 Android Programming In Java: Starting With an App Third Edition
Is now available in paperback and ebook.
Available from Amazon.
- Getting Started With Android Studio 3
- The Activity And The UI
- Building The UI and a Calculator App
- Android Events
Extract: Using Lambdas
- Basic Controls
- Layout Containers
- The ConstraintLayout
Extract: Guidelines and Barriers
- UI Graphics A Deep Dive
Extract: Programming the UI ***NEW
- Menus & The Action Bar
- Menus, Context & Popup
- Resources
- Beginning Bitmap Graphics
Extract: Simple Animation
- Staying Alive! Lifecycle & State
- Spinners
- Pickers
- ListView And Adapters
If you are interested in creating custom template also see:
Custom Projects In Android Studio
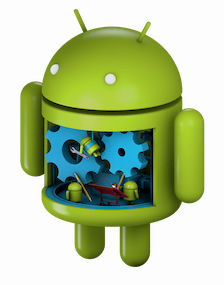
Any small scale data that your app uses - strings, constants, graphics, layout files - should all be included as resources rather than hard coded into your app.
For example, until now we have entered any text that the UI needed directly as a string property value. If you have a Button then this approach is to set the Text property by typing in a string such as "Hello World".
If you run the Warnings and Errors "linter" by clicking on the red icon in the designer then for any string you have entered as a literal into the Properties window you will see a warning:
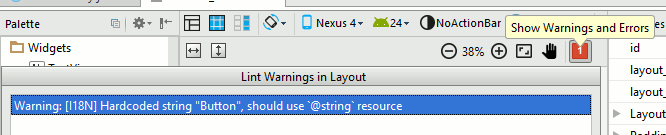
While you shouldn't panic about every warning that the linter shows you this one is a good idea because a resource can be reused in other parts of the program and it is easy to change without having to dive into the code. A much bigger advantage is that you can provide alternative resources which are automatically selected by your app according to the conditions. For example in this case you can provide strings in another language and they will be used automatically according to the locale set when the app runs. This is very a very powerful way to create custom apps that work anywhere in the world and on any device.
So how do we create a resource?
It does depend on the type of the resource but in Android Studio there are two ways of creating a string resource.
The first is to click the "..." at the right of the property entry box which makes the Resources dialog popup.
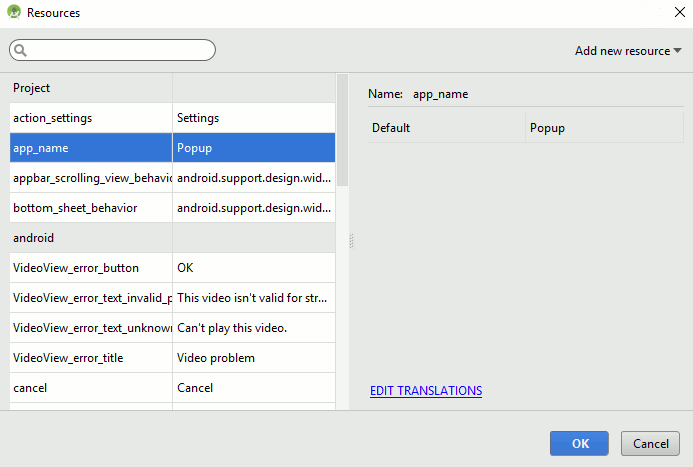
This lists existing resources defined by the system, the project and the theme in use. You can also us the Add new Resource button to create additional resources. In this case a string resource:
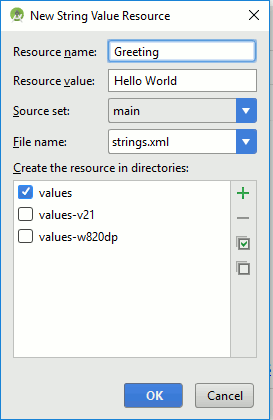
All you have to do is enter a resource name and its value - the rest of the entries can be left at their defaults, their meaning will become clear as we proceed.
When you click OK you will see the Properties window with the entry
@string/Greeting
This is a reference to your newly created resource. You will also see the string that you have specified appear as the button's caption.
You can now reuse the string resource by entering @string/Greeting anywhere the "Hello World" string is required - of course it has to be used with a property that accepts a string.
If you change the definition of a resource then obviously all of its uses are updated.
The second way of creating a string resource is to edit the resource XML file. If you look at the file strings.xml in the res/values directory you will find it contains:
<resources> <string name="app_name">Resources1</string>
<string name="hello_world">Hello world</string> <string name="action_settings">Settings</string> <string name="Greeting">Hello World</string> </resources>
You can see the hello world string that we have just added and some other strings that Android Studio creates automatically for you - including another hello world string which is used in the initial generated UI.
You can edit the resource file directly or you can use the Resources dialog to create and edit existing string resources.
What Are Resources
Resources are any data that your program wants to use.
Resources are compiled into your program's executable ready to be distributed to your end users as part of your app. For a string this doesn't seem particularly useful, after all you could just as easily use a string constant in code, but for other types of resource it can be the only way to get the data included in the apps executable.
For example, drawables - a range of different types of graphics objects - can be included as resources. if you include a gif file as a resource then that gif file is incorporated into your app by the compiler. This means you don't have to provide the gif as a separate file and you don't have to worry about where it is stored. Notice, however, that adding a gif file to your app increases its size.
All resources are stored in folders within the res directory.
The folder name gives the type of the resource and, as we shall see, can specify when the resource is to be used.
You can see a list of resource types in the documentation/ but the commonly used ones are:
Folder Description
drawable/
Any graphic - typically .png, .gif or .jpg but there are a range of other less frequently used type. The rule is - if its in any sense a graphic it belongs in drawable.
layout/
We have been using layouts since we started programming Android and XML layout is a resource complied into your app ready to be used.
menu/
The XML files that specify a menu are also resources and compiled into your app.
values/
XML files that define simple values such as strings, integers and so on. You can think of these as defining constants for your program to use.
Although you can put any mixture of value-defining XML into a single file in the values/ directory, it is usual to group values by data type and or use.
Typically:
- arrays.xml for typed arrays
- color.xml for color values
- dimens.xml for dimensions
- strings.xml for strings
- styles.xml for styles
You can also include arbitrary files and arbitrary XML files in raw/ and xml/. There are two directories dealing with animation animator/ and anim/.
There is also a directory where you can store icons suitable for different screen resolutions, but more of this when we look at conditional resources.
|