Page 1 of 4 The problem with entering the AWS world for the first time is that it's like entering a labyrinth, a futile attempt of navigating through an endless maze of documentation, policies, endpoints, services, tokens and authentications. The task of having to hook all that up in order to get on with your job is daunting. Here is the help you need for AWS Lambda.
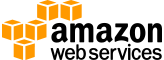 Tthe range of AWS offerings is just immense with so many services and providers to choose from, like EC2, S3, Athena, Kinesis, Lambdas, CloudFront, DynamoDB, API Gateway, and the list goes on.
In this tutorial, however, we're going to take a deep look into the Lambdas and the serverless architecture they support.The beauty of serverless computing isn't that you don't need a server, but you don't need to manage one.Your function lives in its own space on the cloud, capable of being called through multiple entry points, while despite self-contained, still capable of calling other functions or APIs , triggering something akin to a chain reaction:
You can use AWS Lambda to run your code in response to events, such as changes to data in an Amazon S3 bucket or an Amazon DynamoDB table; to run your code in response to HTTP requests using Amazon API Gateway; or invoke your code using API calls made using AWS SDKs. With these capabilities, you can use Lambda to easily build data processing triggers for AWS services like Amazon S3 and Amazon DynamoDB process streaming data stored in Amazon Kinesis, or create your own back end that operates at AWS scale, performance, and security.
Other advantages of using Lambdas, in relation let's say to Alexa but pertaining to any service, can be summarized in:
- You do not need to administer or manage any of the compute resources for your service.
- You do not need an SSL certificate.
- You do not need to verify that requests are coming from the Alexa service yourself. Access to execute your function is controlled by permissions within AWS instead.
- AWS Lambda runs your code only when you need it and scales with your usage, so there is no need to provision or continuously run servers.
- Alexa encrypts its communications with Lambda utilizing TLS. See also the AWS Security Best Practices whitepaper.
- For most developers, the Lambda free tier is sufficient for the function supporting an Alexa skill. The first one million requests each month are free. Note that the Lambda free tier does not automatically expire, but is available indefinitely.
- AWS Lambda supports code written in Node.js (JavaScript), Java, and Python and recently C#, while your client can be coded in anything.
This of course can't be done in isolation since we also need the help of other APIs and services in order to utilize Lambdas, hence in this tutorial we'll also take a look into calling our Lambda functions through API Gateways and HTTP endpoints by using tools such as Postman, Powershell, AWS CLI and finally Perl's Paws module. We're also going to fire authenticated requests tied to IAM Roles and managed policies, understand what's going on behind the scenes by going through the CloudWatch logs, as well as write our server and client code, the first one in Nodejs while the latter in Perl. In order to get started we first have to create an Amazon Developer account.The good thing with that is that you can start right away and for free with the full available range of AWS products and as soon as you're ready to jump into production then you shall be charged on a monthly basis and per usage depending on the number of API calls that took place or the amount of MBs in bandwidth that was consumed. So without any further ado, let's jump into our tutorial that is split into three huge sub-themes, starting out with creating our very first Lambda function and calling it in an unathenticated way. Step 1- Creating our very first Lambda function After creating and logging in to our root/developer account we are immediately presented with a list encompassing the full range of AWS services on offer.
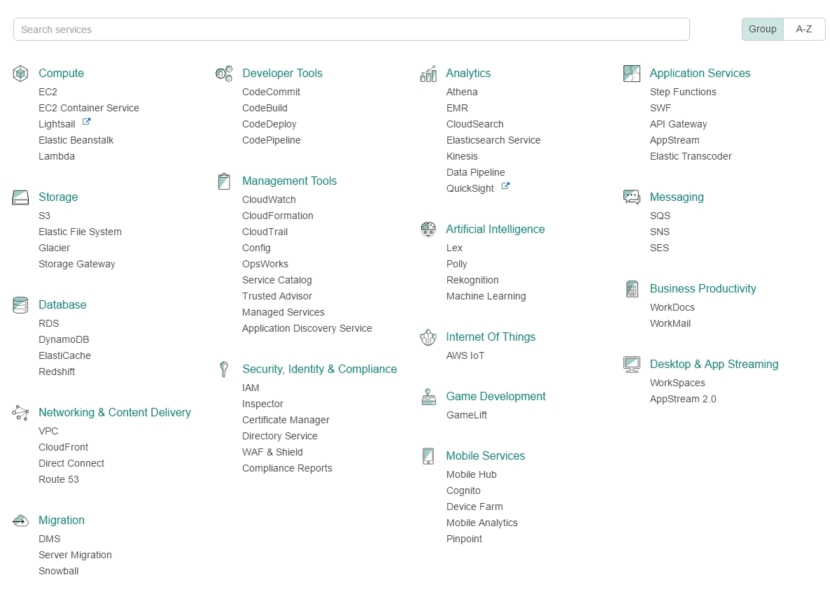
From the list, choose Compute->Lambda, and then from within the presented AWS Lambda dashboard choose Create a Lambda function.  Type hello in the Select blueprint filter to grab the hello-world/Starter AWS Lambda function template. 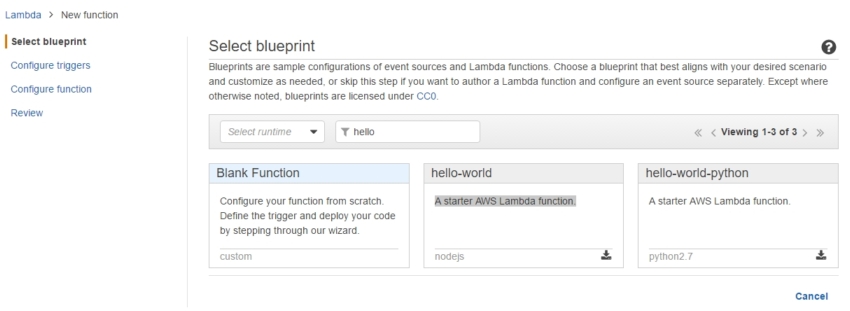 Now it's time to configure our Lambda function, that is choose its runtime infrastructure as Node.js 4.3 and name it as helloWorldNodeJs. 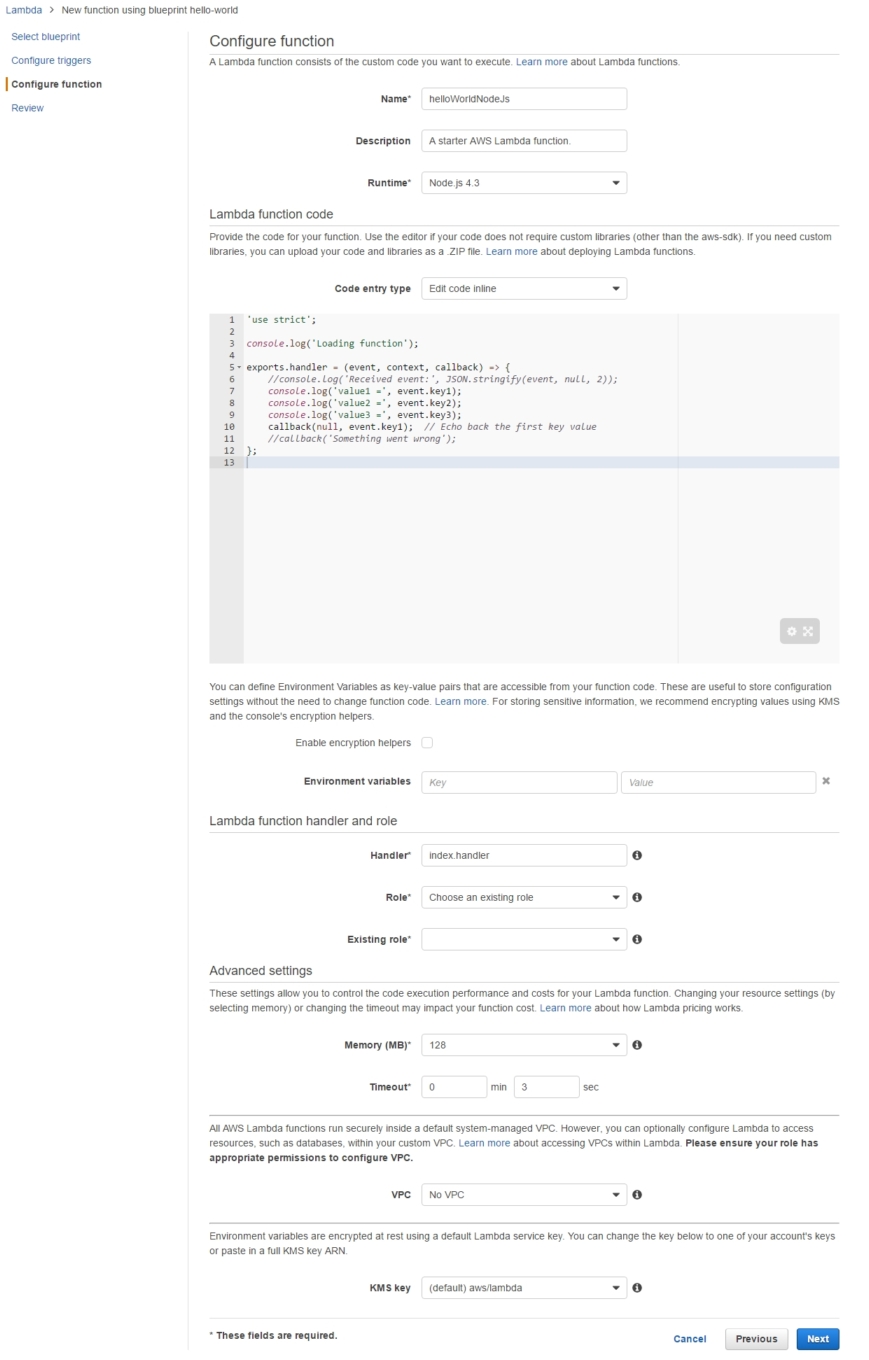 In order to execute a Lambda we have to create a binding with an IAM Role, the most fundamental primitive which should be always active in order to do just about anything on the AWS platform.Its purpose is nicely summarized in:
AWS Lambda uses an IAM role that grants your custom code permissions to access AWS resources it needs
but despite the lengthy description and elaborate wording it's nothing more than the classic Role/User authentication scheme.We can Choose an existing role from the available options but to make the process clearer we're going to just pick Create a custom Role instead.  Name the role as lambda_basic_execution_helloWorldNodeJs and while on it expand the auto-generated policy attached to observe it giving the role permission to access the Logs.That's enough for the time being, but soon enough we'll be adding some more policies/functionality to it, and that highlights yet another way to perceive Roles: as a collection of policies. 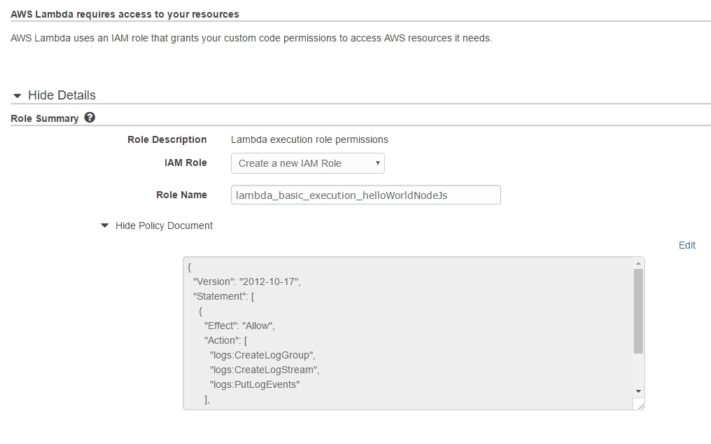 After the role is created we get back to our half-finished Lambda creation dashboard, where the last step remaining is to replace the boilerplate 'hello-world' code with our own:
use strict';
exports.handler = (event, context, callback) => { console.log('Received event:', JSON.stringify(event, null, 2));
var inputObj = JSON.parse(event["body"]); callback(null, {
"statusCode": 200, "headers": { }, // Echo back the first key value "body": JSON.stringify( {"received":inputObj.key1}) })
}
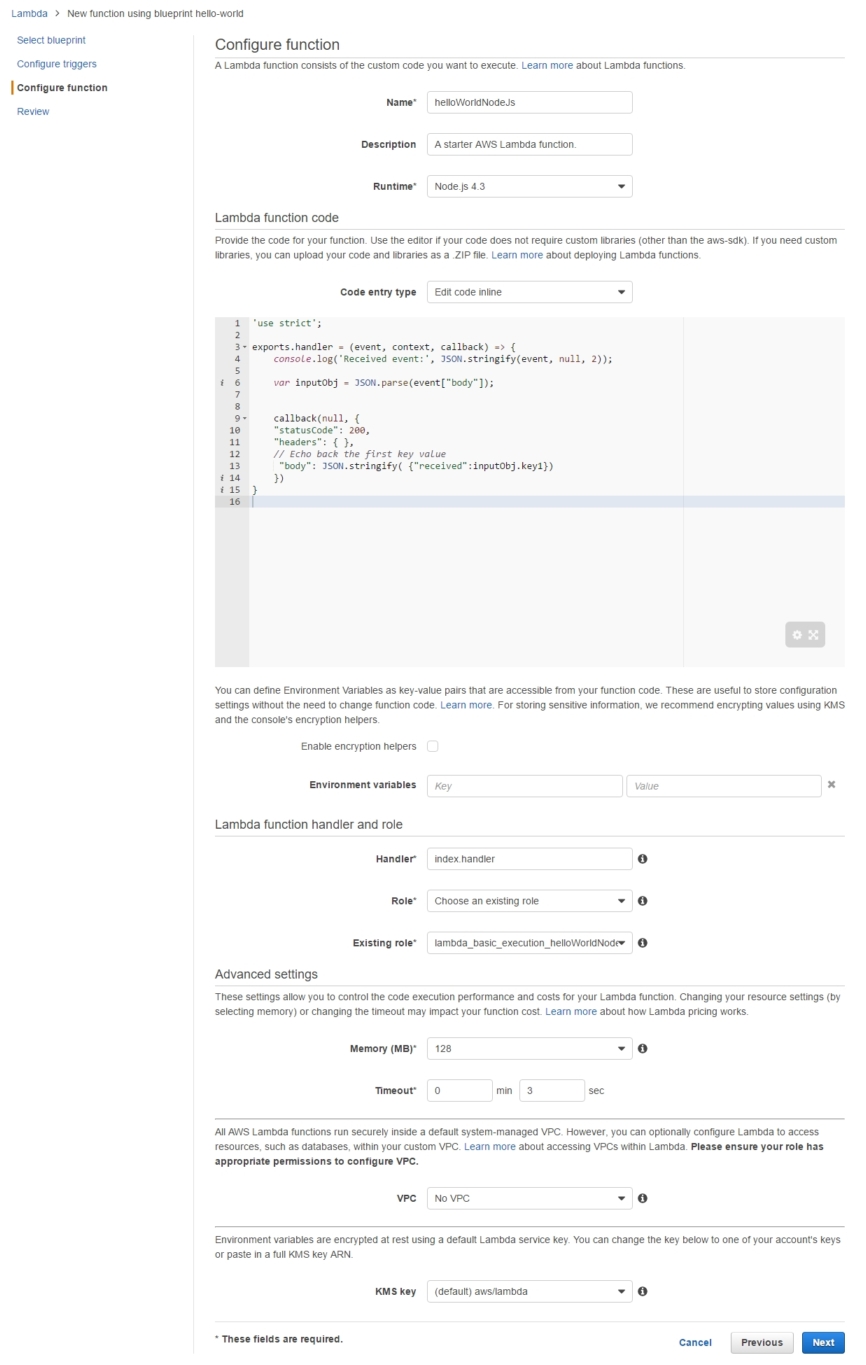
|