Page 2 of 4
The topics covered in Java SE 8 Programmer II are:
- Java Class Design
- Create and use singleton classes and immutable classes
- Develop code that uses static keyword on initialize blocks, variables, methods, and classes
- Implement encapsulation
- Implement inheritance including visibility modifiers and composition
- Implement polymorphism
- Override hashCode, equals, and toString methods from Object class
- Advanced Java Class Design
- Create and use Lambda expressions
- Create inner classes including static inner class, local class, nested class, and anonymous inner class
- Develop code that declares, implements and/or extends interfaces and use the @Override annotation
- Develop code that uses abstract classes and methods
- Develop code that uses final keyword
- Use enumerated types including methods, and constructors in an enum type
- Generics and Collections
- Collections Streams and Filters
- Create and use ArrayList, TreeSet, TreeMap, and ArrayDeque objects
- Create and use a generic class
- Describe Stream interface and Stream pipeline
- Filter a collection by using lambda expressions
- Iterate using forEach methods of Streams and List
- Use java.util.Comparator and java.lang.Comparable interfaces
- Use method references with Streams
- Lambda Built-in Functional Interfaces
- Develop code that uses binary versions of functional interfaces
- Develop code that uses primitive versions of functional interfaces
- Develop code that uses the UnaryOperator interface
- Use the built-in interfaces included in the java.util.function package such as Predicate, Consumer, Function, and Supplier
- Java Stream API
- Develop code that uses Stream data methods and calculation methods
- Develop code that uses the Optional class
- Develop code to extract data from an object using peek() and map() methods including primitive versions of the map() method
- Save results to a collection using the collect method and group/partition data using the Collectors class
- Search for data by using search methods of the Stream classes including findFirst, findAny, anyMatch, allMatch, noneMatch
- Sort a collection using Stream API
- Use flatMap() methods in the Stream API
- Exceptions and Assertions
- Create custom exceptions and Auto-closeable resources
- Test invariants by using assertions
- Use Autoclose resources with a try-with-resources statement
- Use catch, multi-catch, and finally clauses
- Use try-catch and throw statements
- Use Java SE 8 Date/Time API
- Create and manage date-based and time-based events including a combination of date and time into a single object using LocalDate, LocalTime, LocalDateTime, Instant, Period, and Duration
- Define and create and manage date-based and time-based events using Instant, Period, Duration, and TemporalUnit
- Work with dates and times across timezones and manage changes resulting from daylight savings including Format date and times values
- Java I/O Fundamentals
- Read and write data from the console
- Use BufferedReader, BufferedWriter, File, FileReader, FileWriter, FileInputStream, FileOutputStream, ObjectOutputStream, ObjectInputStream, and PrintWriter in the java.io package
- Java File I/O (NIO.2)
- Use Files class to check, read, delete, copy, move, manage metadata of a file or directory
- Use Path interface to operate on file and directory paths
- Use Stream API with NIO.2
- Java Concurrency
- Create worker threads using Runnable, Callable and use an ExecutorService to concurrently execute tasks
- Identify potential threading problems among deadlock, starvation, livelock, and race conditions
- Use java.util.concurrent collections and classes including CyclicBarrier and CopyOnWriteArrayList
- Use parallel Fork/Join Framework
- Use parallel Streams including reduction, decomposition, merging processes, pipelines and performance
- Use synchronized keyword and java.util.concurrent.atomic package to control the order of thread execution
- Building Database Applications with JDBC
- Describe the interfaces that make up the core of the JDBC API including the Driver, Connection, Statement, and ResultSet interfaces and their relationship to provider implementations
- Identify the components required to connect to a database using the DriverManager class including the JDBC URL
- Submit queries and read results from the database including creating statements, returning result sets, iterating through the results, and properly closing result sets, statements, and connections
- Localization
- Build a resource bundle for each locale and load a resource bundle in an application
- Create and read a Properties file
- Read and set the locale by using the Locale object
Are you exam ready?
While the curriculum may seem straightforward the exam questions are recognized to be tricky and can catch out even experienced Java developers, so a good way to discover if you are ready to tackle the OCA exam is to attempt exam questions. In fact, however, much confidence you have as a Java developer doing a practice exam is a good idea in order to familiarize yourself with the multiple choice format.
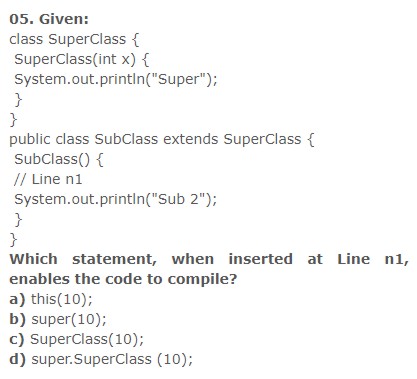
You'll find various sources of sample questions online, including from Oracle itself, but one that caught our attention is Oracle Study. For $26.90 you have 2 months access to 1285+ questions designed to be an exact match to the actual 1Z0-808 exam (940+ questions in the case of 1Z0-809). Each time you attempt the exam questions are selected at random with the multiple choice answers shuffled.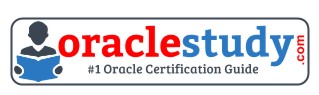
Oracle Study makes the claim that candidates who are able to score 100% in its online practice exam can easily score 80% in the actual exam and student testimonies bear this out, with this from June 2018 being typical:
I practiced for 1Z0-808 Java SE 8 Programmer I every day for a few weeks on oraclestudy.com, I was able to score above 95% during that time. The last two times, I reached to 98% as well. Then I took my actual java certification exam. I found all the questions similar to what I practiced here. I got 84% and passed the exam.
Oracle Study offers 100% money back guarantee for not passing the exam at the first attempt.
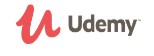
Udemy has several OCA exam preparation options. The most recent, and most highly rated, with 4.6 out of 5 stars is Oracle Java Certification: Shortest Way to Crack1Z0-808.
 
It provides four "TopicWise" tests of 35 questions each that tackle the curriculum in a logical order, the first of them covering Java Features, Data Types, Literals, Arrays and Variables Types. There are also two Grand Tests of 70 questions each. Clear explanations are given for each of the 280 questions.
If you aren't exam ready there are a range of options to get the training you need via a range of methods and here we consider books and training courses, both onsite and online.
|