Page 1 of 3 JavaScript should not be judged as if it was a poor version of the other popular languages - it isn't a Java or a C++ clone. It does things its own way. In particular its functions are objects and this is often missed. This is an extract from JavaScript Jems: The Amazing Parts.
Jem 3
Functions Are Objects
“Form follows function - that has been misunderstood. Form and function should be one, joined in a spiritual union.”
Frank Lloyd Wright
Now available as a book from your local Amazon.
JavaScript Jems: The Amazing Parts
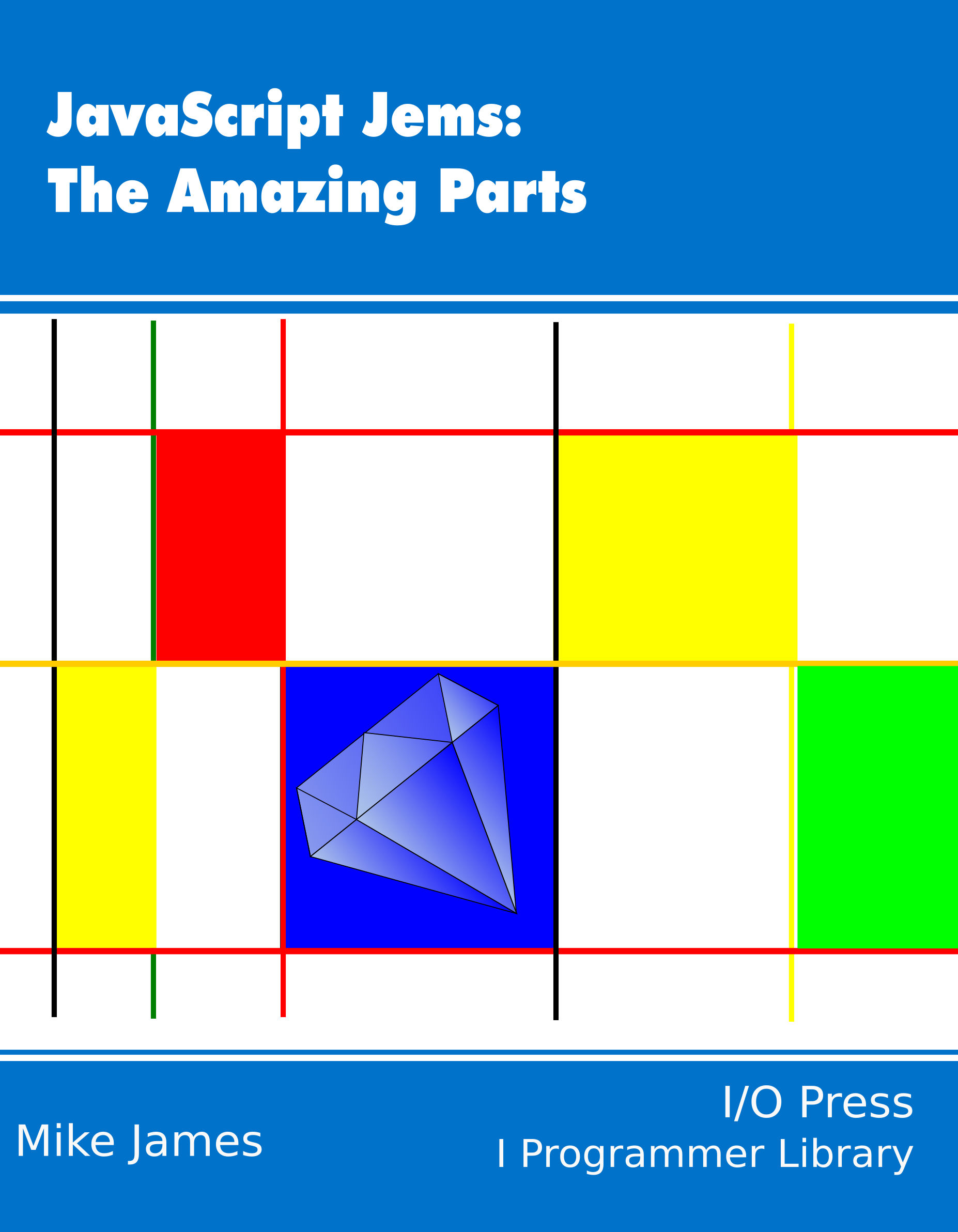
Contents
This jem is just the fact that functions are objects. This is often repeated but little understood. In particular, when it is summarized as “functions are first class objects” it is too easy to miss the full implications and just conclude that you can pass functions as arguments to other functions. This is true, but there is so much more. A better title for this jem would be “Functions are just objects with some code”, but this wouldn’t be understood by all until they had read to the end.
JavaScript, inherently an unusual language, does its best to appear “normal”. So when you first learn about functions you are told to use a “normal” way to define them:
function myFunction(a,b){
var answer=a+b;
return answer;
}
Yes, this looks like a function as you might define it in almost any language with perhaps slight variations in syntax. It looks as if you are defining a function that is just a block of code, but this isn’t the whole story.
JavaScript functions are objects and this is clearly revealed by the alternative way of defining them, the function expression:
var myFunction=function(a,b){
var answer=a+b;
return answer;
}
This is often described as defining an anonymous function, but this is a bit misleading as in JavaScript all functions, and indeed all objects, are anonymous in this sense.
While the first version makes it look as if myFunction is the name permanently allocated to the function, no matter how you create the function you can treat myFunction as a variable and assign a new object to it. In this sense myFunction is not a fixed permanent name for the function.
Note: most JavaScript engines will take note of the name myFunction as assigned in the first version and report debug information about the function using the name – you can still assign to it, however, and break any association between the name and the function.
There is also a full constructor for a Function object;
var myFunction=new Function("a","b", "var result=a+b;return result");
which defines the same Function object as the previous two expressions. There are some small differences but this isn’t important in the present context.
It is important to always think of a function as just an object that happens to have some code that you can execute. That is, myFunction is a reference to an object just like any other and myFunction(parameters) executes the code associated with the object. Of course, the only objects that have code are function objects and so can be executed - they are callable objects.
OK, so a function, even if it sometimes doesn’t look like it, is an object – so what?
There are three large consequences of this and the first is the best known:
-
You can use a function anywhere you can use an object. In particular you can pass a function to another function.
-
Function objects can be created within other objects and so have a lifetime independent of when they are executed and this is the reason for implementing closure.
-
Functions can have properties that exist even when the function is not being executed.
Let’s look at each of these in turn.
|