Page 1 of 3 Classes can do the same job as C# structs - so why both? Find out in this extract from my new book, Deep C#:Dive Into Modern C#.
Deep C#
Buy Now From Amazon
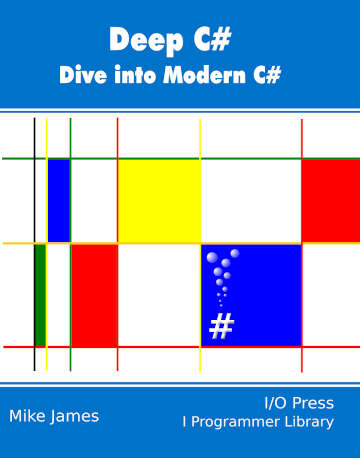
Chapter List
- Why C#?
I Strong Typing & Type Safety
- Strong Typing
Extract Why Strong Typing
- Value & Reference
- Extract Value And Reference
- Structs & Classes
Extract Structs & Classes
- Inheritance
Extract Inheritance
- Interfaces & Multiple Inheritance
Extract Interface
- Controlling Inheritance
II Casting & Generics
- Casting - The Escape From Strong Typing
Extract Casting I ***NEW!
- Generics
- Advanced Generics
- Anonymous & Dynamic
Typing III Functions
- Delegates
- Multicast Delegates
- Anonymous Methods, Lambdas & Closures
IV Async
- Threading, Tasks & Locking
- The Invoke Pattern
- Async Await
- The Parallel For
V Data - LINQ, XML & Regular Expressions
- The LINQ Principle
- XML
- LINQ To XML
- Regular Expressions
VI Unsafe & Interop
- Interop
- COM
- Custom Attributes
- Bit Manipulation
- Advanced Structs
- Pointers
Extra Material
<ASIN:1871962714>
<ASIN:B09FTLPTP9>
Most languages have something like the C# struct – they may call it something different, often a “record”, but the idea is the same. A struct is a collection of different data types with each data type beginning identified as a “field” – similar to a name and address card or record. C# has structs but they play a deeper role in the language being more like classes than the simple data structures they are in other languages.
Structs Are Value Types
Although value types are often introduced as “simple” types such as int or float , all value types are really just examples of struct
which is generally thought of as a more complicated type with multiple values as fields.
The simple value types are structs, but they are also treated differently to avoid the overheads a genuine struct brings with it to make sure that your program runs efficiently. The fact that an int is a struct really only has an impact on your programs because this means that int inherits a set of simple standard methods from object . For example, it is perfectly OK to write:
int
a;
string b = a.ToString();
In fact, int is just an alias for the System.Int32 struct and you could write:
System.Int32
a;
in place of int
a , but it is usual not to. We will return to the issue of simple data types as objects later in this chapter because there is a little more to it.
It is reasonable to say that the most important division in the C# type system is the split into classes and structs (both descended from object ). And the really big difference between the two is that class is a reference type whereas struct is a value type.
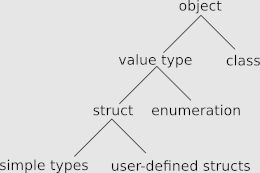
Structs are from value and classes are from reference
In many cases you have the choice of implementing something either as a class or a struct . For example consider a simple type designed to store the x,y coordinates of a point.
You can do this as a class :
c lass
PointR
{
public int x,y;
}
or as a struct :
s truct
PointV
{
public int x,y;
}
Notice that the class is named with a trailing R for Reference and the struct with a trailing V for value.
The most important difference is due to the fact that a struct is a value type and a class is a reference type. That is, the class behaves as described earlier for general reference types and struct behaves like a general value type.
|