Page 3 of 4
Step 3 - Hooking up the Actions Back to our API Gateway and our LambdaMicroServiceHelloWorldNodeJS API.This time we are going to define the HTTP methods that our API will listen to as well as how to react to their invocation.As Lambdas work only with POST requests, we add just the POST method: Actions->Create Method->POST->POST Setup Integration Type:HTTP Endpoint URL:https://bwxuad56tl.execute-api.eu-west-1.amazonaws.com/prod/helloWorldNodeJs
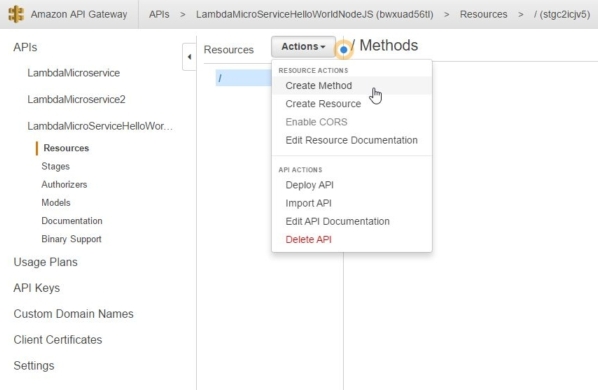 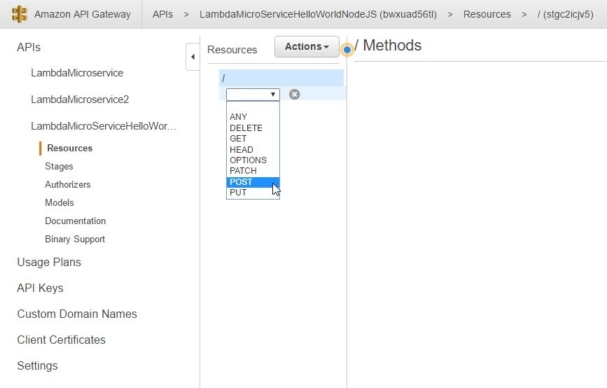 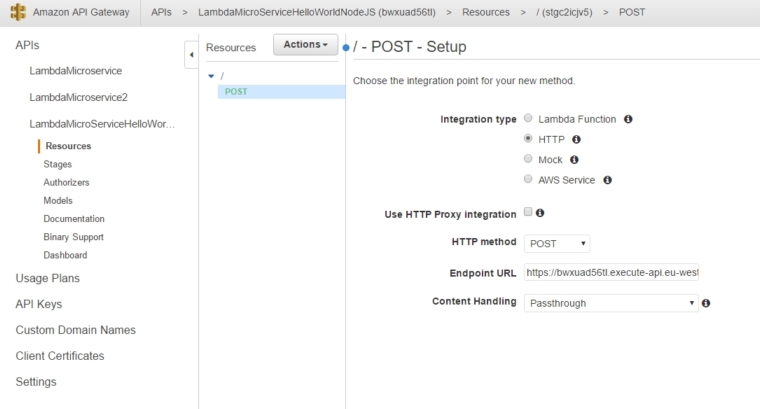 If all goes well we should be presented with a screen similar to: 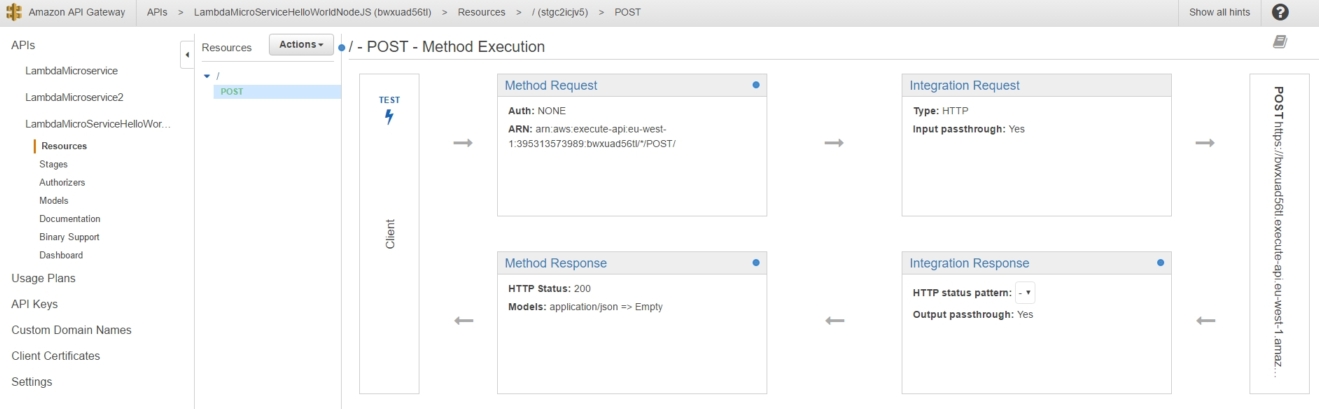 Step 4 - The real test with Postman Download the Postman Chrome extension.The nice thing with it is that Postman can be also installed as a standalone desktop app, so go ahead and make that choice when prompted for it. Now from within Postman pick POST as the method and enter our URL endpoint to the input box next to it: https://bwxuad56tl.execute-api.eu-west-1.amazonaws.com/prod/helloWorldNodeJs
As for Authorization pick No Auth 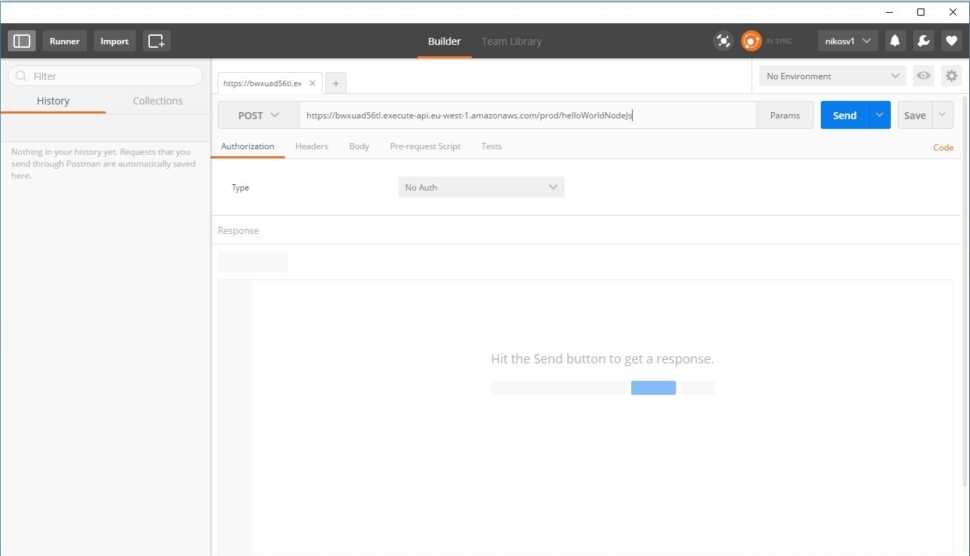
On the Headers tab enter Content-Type application/json 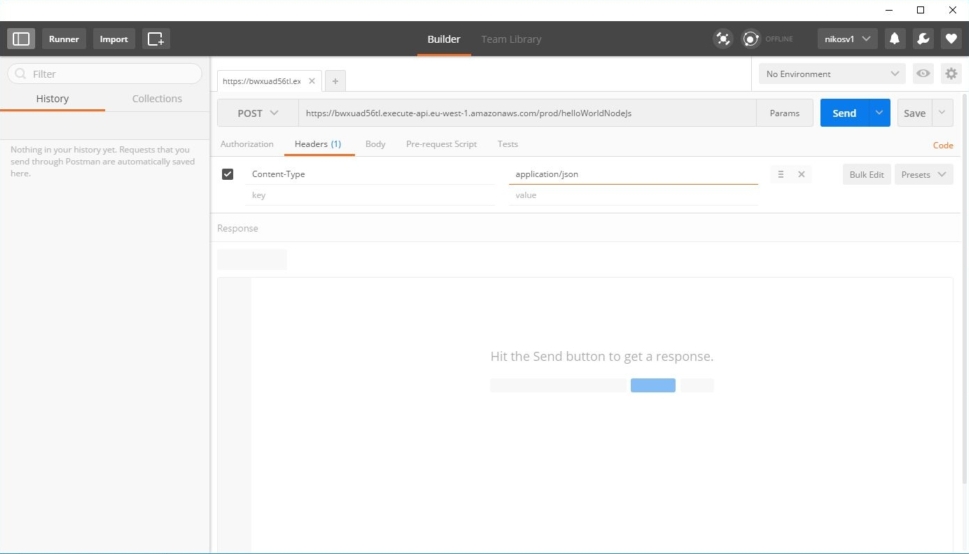 On the Body tab, choose raw and type {"key1":"value1"} as the payload which is going to be forwarded through our endpoint to our backend Lambda function.
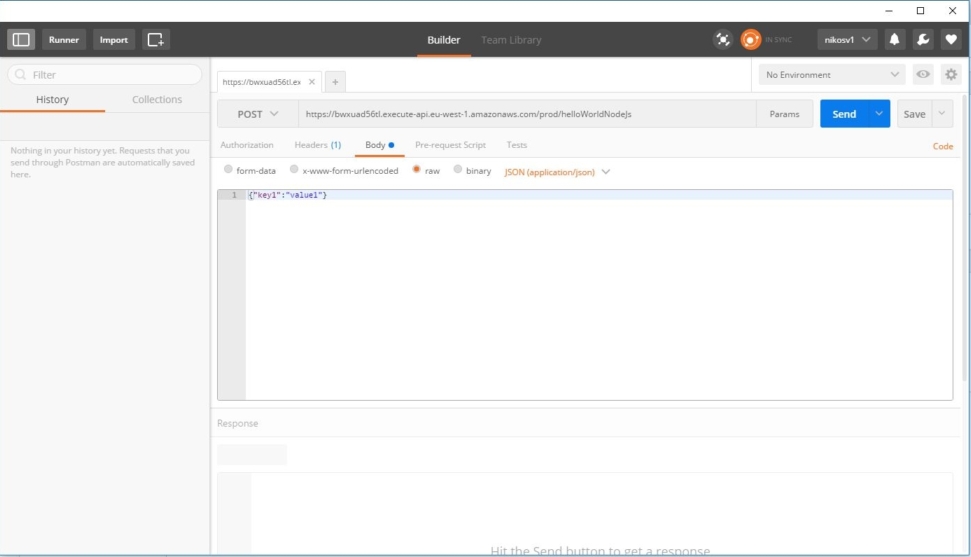 Finally, push Send and watch Postman receive a successful (hopefully) reply that echoes back the value sent the function:
{
"received":"value1"
}
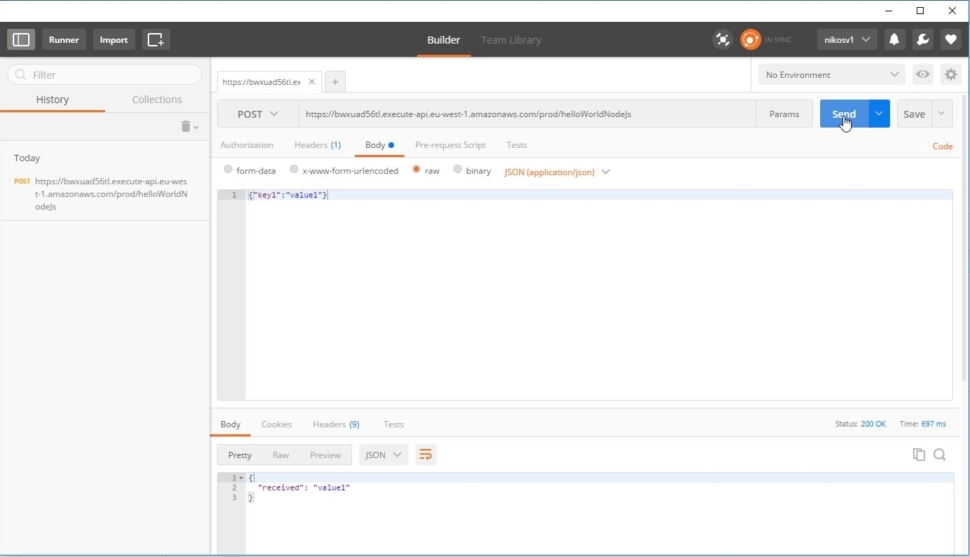 Let's look in detail what our function did:
'use strict';
exports.handler = (event, context, callback) => {
console.log('Received event:', JSON.stringify(event,
null, 2));
var inputObj = JSON.parse(event["body"]);
callback(null, {
"statusCode": 200,
"headers": { },
// Echo back the first key value
"body": JSON.stringify(
{"received":inputObj.key1})
})
}
Seems that the call to our trigger fired an event that encapsulated the POST request's body, that is our JSON string {"key1":"value1"} , which among others, got forwarded to our function.
After parsing the string into a JSON object, we call it's key1 property through inputObj.key1 which replies with value1 . We pack that reply into a JSON string with {"received":inputObj.key1} and push it back to our client, Postman in this case.
As a matter of fact let's change client and see if the same call works through Powershell
$json = "{
""key1"" :
""value1""
}"
Invoke-WebRequest -Uri
https://bwxuad56tl.execute-api.eu-west-1.amazonaws.com/prod/helloWorldNodeJs
-usebasicparsing -Method POST -ContentType "application/json" -Body $json
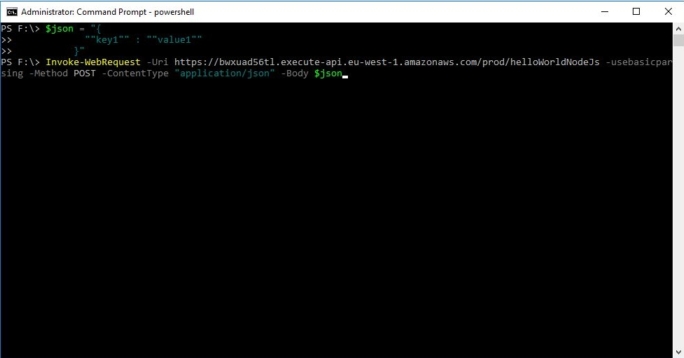
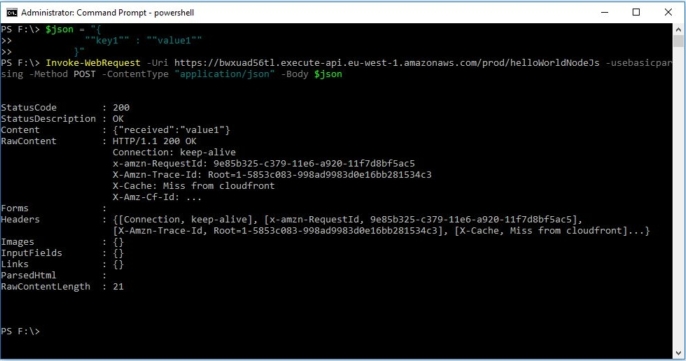
|