Page 3 of 4
Step 5 - Identify and locate the dependencies The problem now is how to find out which libraries our client depends on as well as locating them from within the Oracle Maven repository, which remember cannot be browsed.
Fortunately the Maven Core Indexer is packed as a gzipped file and available at https://maven.oracle.com/.index/nexus-maven-repository-index.gz.
Another alternative way in navigating the index is through an IDE like NetBeans which has a Maven repository browser build in or with the Nexus Repository OSS manager, but I'll forgo both of them because as promised I'll do everything from the CLI.
So downloading and unzipping 'nexus-maven-repository-index.gz' extracts the 'nexus-maven-repository-index' file which can be opened in a plain text editor and, although not a feast for the eyes, is sufficient for the job.
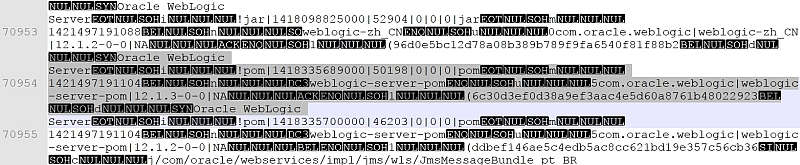
Now we must search through this file to locate the necessary dependencies.Of course we need the core weblogic-server library and its version edition hosted on the repo, that is '12.1.3-0'.
Next we pick the code's import statements apart, such as import weblogic.xml.crypto.wss.WSSecurityContext and look for the package hosting the WSSecurityContext class.The corresponding entry inside the index file is /weblogic/xml/crypto/wss/WSSecurityContext.
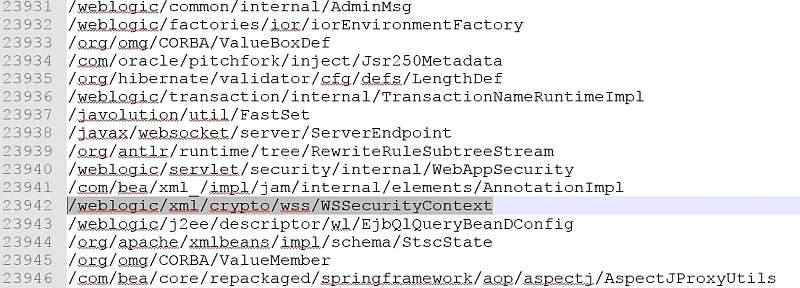
which is found in several jars such as wlnmclient.jar, wljmxclient.jar and wlsafclient.jar.Since the subsequent searches for the rest of the classes reveals that most of them are found inside wlsafclient it was the one picked, that way avoiding getting dragged into the 'find the right library' going through the convoluted official manual.
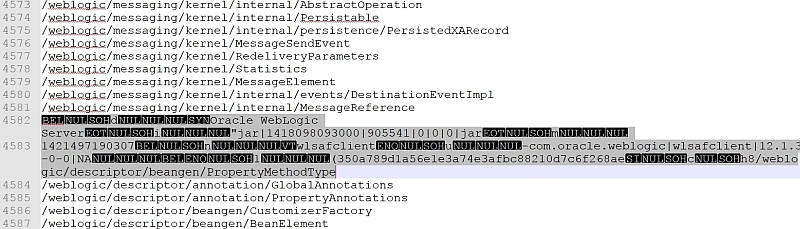
Another alternative is to use JarScan which goes to reveal in which jar file a particular class resides thus resolving any NoClassDefFoundError, ClassDefNotFound, and ClassNotFoundException problems caused by missing classes.
Step 6 - Amend the project's pom file After locating the necessary dependencies we have to incorporate them into the project's pom file.But what is a POM file?
A Project Object Model or POM is the fundamental unit of work in Maven. It is an XML file that contains information about the project and configuration details used by Maven to build the project. It contains default values for most projects. Examples for this is the build directory, which is target; the source directory, which is src/main/java; the test source directory, which is src/test/java; and so on.
So let's amend pom.xml and add the following:
<dependencies> <dependency> <groupId>com.oracle.weblogic</groupId> <artifactId>weblogic-server-pom</artifactId> <version>12.1.3-0-0</version> <type>pom</type> <scope>compile</scope> </dependency> <dependency> <groupId>com.oracle.weblogic</groupId> <artifactId>wlsafclient</artifactId> <version>12.1.3-0-0</version> <scope>compile</scope> </dependency> </dependencies>
This will pull the dependencies together and make them available to our App.java application. But in order to also bundle them into the final fat jar we must instruct maven to use the 'maven-assembly-plugin' :
<build> <finalName>wlogicclient</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-assembly-plugin</artifactId> <version>2.4.1</version> <configuration> <!-- get all project dependencies --> <descriptorRefs> <descriptorRef>jar-with-dependencies</descriptorRef> </descriptorRefs> <archive> <manifest> <mainClass>com.wlogic.client.App</mainClass> </manifest> </archive> </configuration> <executions> <execution> <id>make-assembly</id> <!-- bind to the packaging phase --> <phase>package</phase> <goals> <goal>single</goal> </goals> </execution> </executions> </plugin> </plugins> </build>
This last action completes our pom.xml file.For the complete listing check the project on GitHub.
Step 7 - Compile the project It's time to tell Maven to compile the project with:
$ mvn -X compile
which makes the actual call to the online repositories, fetches the libraries and caches them into our local repository under the current user's .m2 directory:
[DEBUG] === PROJECT BUILD PLAN ================================================ [DEBUG] Project: com.wlogic.client:wlogicclient:1.0-SNAPSHOT [DEBUG] Dependencies (collect): [] [DEBUG] Dependencies (resolve): [compile] [DEBUG] Repositories (dependencies): [Oracle_Maven_Repo (https://maven.oracle.com, default, releases), central (https://repo.maven.apache.org/maven2, default, releases)] [DEBUG] Repositories (plugins) : [maven.oracle.com (https://maven.oracle.com, default, releases+snapshots), central (https://repo.maven.apache.org/maven2, default, releases)] [DEBUG] ----------------------------------------------------------------------- [DEBUG] Goal: org.apache.maven.plugins:maven-resources-plugin:2.6:resources (default-resources) [DEBUG] Style: Regular DEBUG] ======================================================================= [DEBUG] Using transporter WagonTransporter with priority -1.0 for https://maven.oracle.com [DEBUG] Using connector BasicRepositoryConnector with priority 0.0 for https://maven.oracle.com with username=***, password=*** via proxy.myorg.com:8080 with username=, password=*** . . Downloading: https://maven.oracle.com/junit/junit/3.8.1/junit-3.8.1.pom . . Downloading: https://maven.oracle.com/com/oracle/weblogic/weblogic-server-pom/12.1.3-0-0/weblogic-server-pom-12.1.3-0-0.pom . . [DEBUG] Writing tracking file /home/test/.m2/repository/com/oracle/weblogic/weblogic-server-pom/12.1.3-0-0/_remote.repositories [DEBUG] Writing tracking file /home/test/.m2/repository/com/oracle/weblogic/weblogic-server-pom/12.1.3-0-0/weblogic-server-pom-12.1.3-0-0.pom.lastUpdated [DEBUG] Could not find metadata com.oracle.weblogic:weblogic/maven-metadata.xml in local (/home/test/.m2/repository)
And so on until we get to :
[INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 24:08 min [INFO] Finished at: 2017-08-30T07:15:30+03:00 [INFO] Final Memory: 37M/77M [INFO] -------
Completing the compilation phase adds a few more files to our project structure too:
wlogicclient
|-- pom.xml
|-- src
| |-- main
| | `-- java
| | `-- com
| | `-- wlogic
| | `-- client
| | `-- App.java
| `-- test
| `-- java
| `-- com
| `-- wlogic
| `-- client
| `-- AppTest.java
`-- target
|-- classes
| `-- com
| `-- wlogic
| `-- client
| `-- App.class
`-- maven-status
`-- maven-compiler-plugin
`-- compile
`-- default-compile
|-- createdFiles.lst
`-- inputFiles.lst
20 directories, 6 files
|