Page 1 of 3 In this chapter extract the aim is to show how Function objects are used as methods by other JavaScript objects. Methods aren't just functions, they are functions that work with the object they are bound to. Find out how Functions become methods.
This is an extract from the book Just JavaScript by Ian Elliot.
Buy Now: from your local Amazon
Just JavaScript An Idiomatic Approach
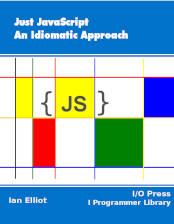
A Radical Look At JavaScript
Most books on JavaScript either compare it to the better known class based languages such as Java or C++ and even go on to show you how to make it look like the one of these.
Just JavaScript is an experiment in telling JavaScript's story "just as it is" without trying to apologise for its lack of class or some other feature. The broad features of the story are very clear but some of the small details may need working out along the way - hence the use of the term "experiment". Read on, but don't assume that you are just reading an account of Java, C++ or C# translated to JavaScript - you need to think about things in a new way.
Just JavaScript is a radical look at the language without apologies.
Contents
- JavaScript – Essentially Different
- In The Beginning Was The Object
- Real World Objects
- The Function Object
Extract - The Function Object Extract - Function Object Self Reference
- The Object Expression
- Function Scope, Lifetime & Closure
Extract Scope, Lifetime & Closure Extract Execution Context ***NEW!
- Parameters, Returns and Destructuring
Extract - Parameters, and Destructuring
- How Functions Become Methods
- Object Construction
Extract: - Object Factories
- The Prototype
Extract - ES2015 Class and Extends
- Inheritance and Type
- The Search For Type
- Property Checking
Buy Now: from your local Amazon
Also by Ian Elliot JavaScript Async: Events, Callbacks, Promises and Async Await Just jQuery: The Core UI Just jQuery: Events, Async & AJAX
<ASIN:1871962579>
<ASIN:1871962560>
<ASIN:1871962501>
<ASIN:1871962528>
So far we have looked at the role that the Function object plays in bringing executable code to JavaScript in general. Other languages have functions, methods or subroutines, but JavaScript has the Function object which is a general object which has an additional default "code property" that can be evaluated using the evaluation or invocation operator ().
What Is A Method?
Before we look at how JavaScript deals with methods it is worth spending a few minutes looking at the general problem.
When we first started writing programs in higher level languages best practice was to write a function for what ever you needed to do.
For example, if you needed to sort an array you would write a sort function which accepted a few parameters that determined the data and the operation:
sort(myArray,order);
Where myArray is the data that you want to sort and order is a parameter that set the sort order to be used.
Later on we moved over to object oriented programming where data and the functions that process the data are grouped together into entities called objects. In this case the functions like sort became methods of the data that they were to operate on.
So an Array object would have a sort method and you would write the sort operation as:
myArray.sort(order);
You can see that this is a small change from the use of myArray as a parameter to the sort function to myArray as an object and sort method. You could say that the whole of the shift from functions to object oriented programming is all about the shift of a parameter from inside the function to outside.
Looking a little deeper the simplification that this shift brings about is well worth it.
The sort function can now use the data in myArray more or less by default and this makes it possible to create an isolation from the rest of the program. It also brings about a complete change in the way that we think about functions.
For example, you could say that myArray “knows how” to sort itself. Another object myList say may also “know how” to sort itself using its own sort function which isn’t the same as the Array sort function.
This means that each data structure can have its own sort function and we can avoid having to have an arraySort function and a listSort function and a…
This is a limited form of polymorphism and it is one of the huge advantages of object oriented programming. Yet it is strange that even the most enthusiastic object oriented programmers don’t think that there is any problem in having overloaded functions to deal with different data types which is a problem much better solved by polymorphism. It is much better to have the data determine which function to use rather than the signature of a detached function.
Now let’s look at the different ways that objects and functions work together in JavaScript.
Objects with function properties
As we discovered in Chapter 2, an object is a set of properties with object values. As a function is nothing more than a Function object there is no reason why a function cannot be a property of another object.
For example:
var myObject={ myFunction: function(){alert("My Function");}};
creates a new object with a single property myFunction which is a Function object with code body:
alert("My Function");
The only problem with defining Function properties is how to format them on the page to make the code clear.
Most use something like:
var myObject={
myFunction: function(){
alert("My Function");
} };
To execute the code body of the Function property you simply need a reference to the Function object and the property i.e.
myObject.myFunction
and the evocation operator () which gives:
myObject.myFunction();
And it is always worth remembering the difference between the two.
If there were parameters they would be included in the usual way between the parentheses.
You can also write:
myObject["myFunction"];
as the reference to the Function object and
myObject["myFunction"]();
as the evaluation of the function body. In this form it looks a lot less like a traditional function call but there is nothing new here either.
Why would you use an object with Function properties?
Namespaces
An object is a good way to gather together functions that do the same sort of job.
For example the built in Math object provides a place to group mathematical functions like sin, cos and so on. To use such a function you have to write something like:
var ans=Math.sin(0.5);
In this case it might seem inconvenient to have to write the full "dotted" reference to the Function object but in general it is useful to be able to separate the name applied to this function and any other function which might happen to have the same name.
In other words using Functions within objects is a way of avoiding name collisions.
When ever you are working on a large program trying to find unique names for things becomes increasingly difficult as the program gets bigger. You generally end up applying a good name to the first function or variable of a particular type such as totalHours only later to have to use something less meaningful such as tHours to avoid the name collision with the first function or variable.
In other languages name collisions are made less of a problem by they use of "namespaces" which assign multipart names for things without having to get involved in creating objects necessarily. Usually the first part of the name is derived by the IDE or the language conventions unless there is a name collision when you have to provide a fully qualified name.
Object are often used in JavaScript as namespaces just like the Math object. If you want to create an alternative function called sin then you can because the built-in sin's full name is Math.sin.
Of course to avoid a name collision with someone else's sin function you would be well advised to put your new function within a suitable object - e.g. myMathFunctions. Of course the trick here is finding a name for your "namespace" object that is going to be unique and so avoid an initial name collision. This is the reason why some programs make use of a domain name for the object e.g. iprogrammerinfo but this is usually unnecessary and makes things look complicated.
You can also make use of multiple nested object to create a nested namespace. So you could have
myMath.trig.sin
or
myMath.optimize.linear
and so on.
JavaScript doesn't have explicit support for namespaces and so using objects as namespaces makes good sense.
You can even simplify naming where required by assigning the fully qualified name to something shorter. For example:
var sin=Math.sin;
and following this statement you can just write
var result=sin(0.5);
To labor the point for one final time; notice that Math.sin isn't a function it is a reference to a Function object that is one of the properties of the Math object.
|