Page 1 of 2 It would be nice if all there was to say about JavaScript objects was the ideas introduced in Chapter two. In fact there are various facilities that have been introduced to make objects more usable. While these don’t change the basic philosophy of JavaScript they can cloud the issue when you first start learning the language. In this chapter we look at some features that make real world objects easier to use.
This is an extract from the book Just JavaScript by Ian Elliot.
Buy Now: from your local Amazon
Just JavaScript An Idiomatic Approach
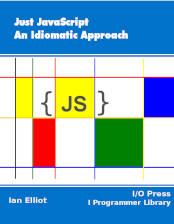
A Radical Look At JavaScript
Most books on JavaScript either compare it to the better known class based languages such as Java or C++ and even go on to show you how to make it look like the one of these.
Just JavaScript is an experiment in telling JavaScript's story "just as it is" without trying to apologise for its lack of class or some other feature. The broad features of the story are very clear but some of the small details may need working out along the way - hence the use of the term "experiment". Read on, but don't assume that you are just reading an account of Java, C++ or C# translated to JavaScript - you need to think about things in a new way.
Just JavaScript is a radical look at the language without apologies.
Contents
- JavaScript – Essentially Different
- In The Beginning Was The Object
- Real World Objects
- The Function Object
Extract - The Function Object Extract - Function Object Self Reference
- The Object Expression
- Function Scope, Lifetime & Closure
Extract Scope, Lifetime & Closure Extract Execution Context ***NEW!
- Parameters, Returns and Destructuring
Extract - Parameters, and Destructuring
- How Functions Become Methods
- Object Construction
Extract: - Object Factories
- The Prototype
Extract - ES2015 Class and Extends
- Inheritance and Type
- The Search For Type
- Property Checking
Buy Now: from your local Amazon
Also by Ian Elliot JavaScript Async: Events, Callbacks, Promises and Async Await Just jQuery: The Core UI Just jQuery: Events, Async & AJAX
<ASIN:1871962579>
<ASIN:1871962560>
<ASIN:1871962501>
<ASIN:1871962528>
Advanced Objects – Expression Value and Key
Later on you will discover that there is a general rule that anywhere you can use an object you can use an object expression.
What this means in this case is that the object that is the value of a property can be produced by an expression.
For example:
{object1:1+2}
creates a property called object1 with the value numeric object 3.
In ES2015 you can also use variables to create properties. In this case the name of the variable is the name of the property and its value its value.
For example:
var object1=1+2;
var object2={};
{object1,object2};
creates an object with properties object1:3 and object2:{}.
Also in ES2015 you can use an expression to set both the name and the value of a property. For example:
{[“myproperty”+n]:n}
assuming n is set to 1 this creates an object with the property myproperty1:1.
Advanced Property Access
Since ES2015 you have been able to define computed properties using get and set. If you are familiar with other object oriented languages you might already be familiar with the idea of getters and setters. Instead of a direct access to a property a get function is automatically called on access and a set function on assignment.
For example:
var myObject={
value:0, get myValue() {return this.value;}, set myValue(v) {this.value=v;} };
myObject.myValue=10;
alert(myObject.myValue);
In this case the object has a property called myValue in addition to value. Notice that the name of the property is myValue and not getmyValue or setmyValue. When you assign to myValue the set function is automatically called with the value assigned. In this case the call sets the “normal” property value to 10. When myValue is accessed as in the alert function a call to the get function automatically occurs to supply the value. Notice that in this case value is a publicly accessible property – you can create a private property but this relies on using a constructor.
Also notice the use of this.value to refer to the value property of the object. This is the call context and will be discussed in more detail in Chapter Eight.
The main use of get and set is often said to be to compute a property from other properties.
For example, area derived from a length and width property.
In most cases however the main use of set is to verify that the property value is valid.
For example if you have a property which is a percentage then you can write:
var myObject={
value:0,
get myValue() {return this.value;}, set myValue(v) {
if(v<0) return; if(v>100) return; this.value=v; } };
The main use of get is to convert an internal representation of a value into something more useful.
For example you might have a library that works with days of the week coded as 0 to 6 but prefer to expose this as the more usual 1 to 7:
var myObject={ day:0, get myValue() {return this.day+1;}, set myValue(v) { if(v<1) return; if(v>7) return; this.day=v-1; } };
This is a fairly trivial example but the general principle is that
|