Page 1 of 2 Object creation is fundamental to all object-oriented languages, but in JavaScript it is left to the programmer to work out how best to do it, and often the practice that you encounter isn't the best because it's borrowed from other languages. In this extract from my recent book the emphasis is on object factories rather than the more familiar constructor.
This is an extract from the book Just JavaScript by Ian Elliot.
Buy Now: from your local Amazon
Just JavaScript An Idiomatic Approach
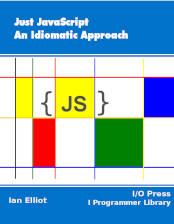
A Radical Look At JavaScript
Most books on JavaScript either compare it to the better known class based languages such as Java or C++ and even go on to show you how to make it look like the one of these.
Just JavaScript is an experiment in telling JavaScript's story "just as it is" without trying to apologise for its lack of class or some other feature. The broad features of the story are very clear but some of the small details may need working out along the way - hence the use of the term "experiment". Read on, but don't assume that you are just reading an account of Java, C++ or C# translated to JavaScript - you need to think about things in a new way.
Just JavaScript is a radical look at the language without apologies.
Contents
- JavaScript – Essentially Different
- In The Beginning Was The Object
- Real World Objects
- The Function Object
Extract - The Function Object Extract - Function Object Self Reference
- The Object Expression
- Function Scope, Lifetime & Closure
Extract Scope, Lifetime & Closure Extract Execution Context ***NEW!
- Parameters, Returns and Destructuring
Extract - Parameters, and Destructuring
- How Functions Become Methods
- Object Construction
Extract: - Object Factories
- The Prototype
Extract - ES2015 Class and Extends
- Inheritance and Type
- The Search For Type
- Property Checking
Buy Now: from your local Amazon
Also by Ian Elliot JavaScript Async: Events, Callbacks, Promises and Async Await Just jQuery: The Core UI Just jQuery: Events, Async & AJAX
One of the most misunderstood parts of JavaScript is the way that objects are created. The reason is that JavaScript doesn't make use of the idea of class, and programmers coming from other languages try to re-implement it. In a language that uses only object instances, reinventing class is a bit of a waste of time.
A class facility was introduced in ES2015, but this is only a very light wrapper for one of the ways of creating new instances with a form of inheritance. You can do exactly the same thing in ES5 without using the new facilities. The ES2015 approach is described in detail in Chapter 10 see ES2015 Class and Extends.
Also the idea that JavaScript is a “prototype-based” language is also misleading. The prototype is often introduced as a way of creating object hierarchies as in a class-based language, but it has a much simpler purpose.
So how do we create objects in JavaScript?
If you already know about JavaScript constructors read on, because how they are arrived at is an important story.
The Classical Constructor
It might help to look first at how class-based languages deal with the problem of creating instances. If you don't know about how class-based languages work, just skip to the next section.
In class-based languages, instances of a class are generally created using the constructor, which implicitly uses the class definition to manufacture an instance of the class.
For example, in language like Java, C++ or C# you would write:
MyClass myObject = new myClass();
where myClass() is the constructor.
The constructor is a function that returns an instance of the class.
This is a fact that is often overlooked by programmers in class-based languages because they don't actually have to write anything in the constructor for it to do its job. That is, an empty or default constructor will automatically do the job of creating an instance. This results in programmers thinking that constructors are really only needed when something has to be initialized or set up in some way.
The idea of a constructor can be extended to the idea of an object factory. An object factory is an object that will create another object – perhaps another instance of its own type or another completely different type.
The idea of a function which creates objects is core to class-based languages and, in fact, to all object-oriented languages.
The JavaScript Object Factory
If you just want a single object then you could simply make use of an object literal and define your object.
For example:
var myObject={prop1:object1,prop2:object2... }
This works perfectly well and it is a good way to make a singleton object, i.e. an object that there should only be one instance of, in any given program. Surprisingly, singletons are the most common case of objects in most programs.
For example, if you want to store the position of a single 2D point you might define:
var myPoint={x:0,y:0};
However, if you want more than one copy of an object you need to automate the creation or implement a method of cloning an existing object. Although cloning is a perfectly proper way to create new instances of objects, it isn't the most usual way of going about the task. In JavaScript the standard practice is to use an object factory, and to be more precise a constructor. Let's take a look at the object factory approach first and then see how it specializes into a constructor.
An object factory is any function that returns a single object as its result. As a function in JavaScript is just an executable object, it sometimes helps to think of an object factory as an object that creates an instance of another object.
How do object factories work?
|