Page 1 of 3 Not every comma in JavaScript is an operator - in fact most of them aren't. It is time to confront the confusing comma.
Now available as a book from your local Amazon.
JavaScript Jems: The Amazing Parts
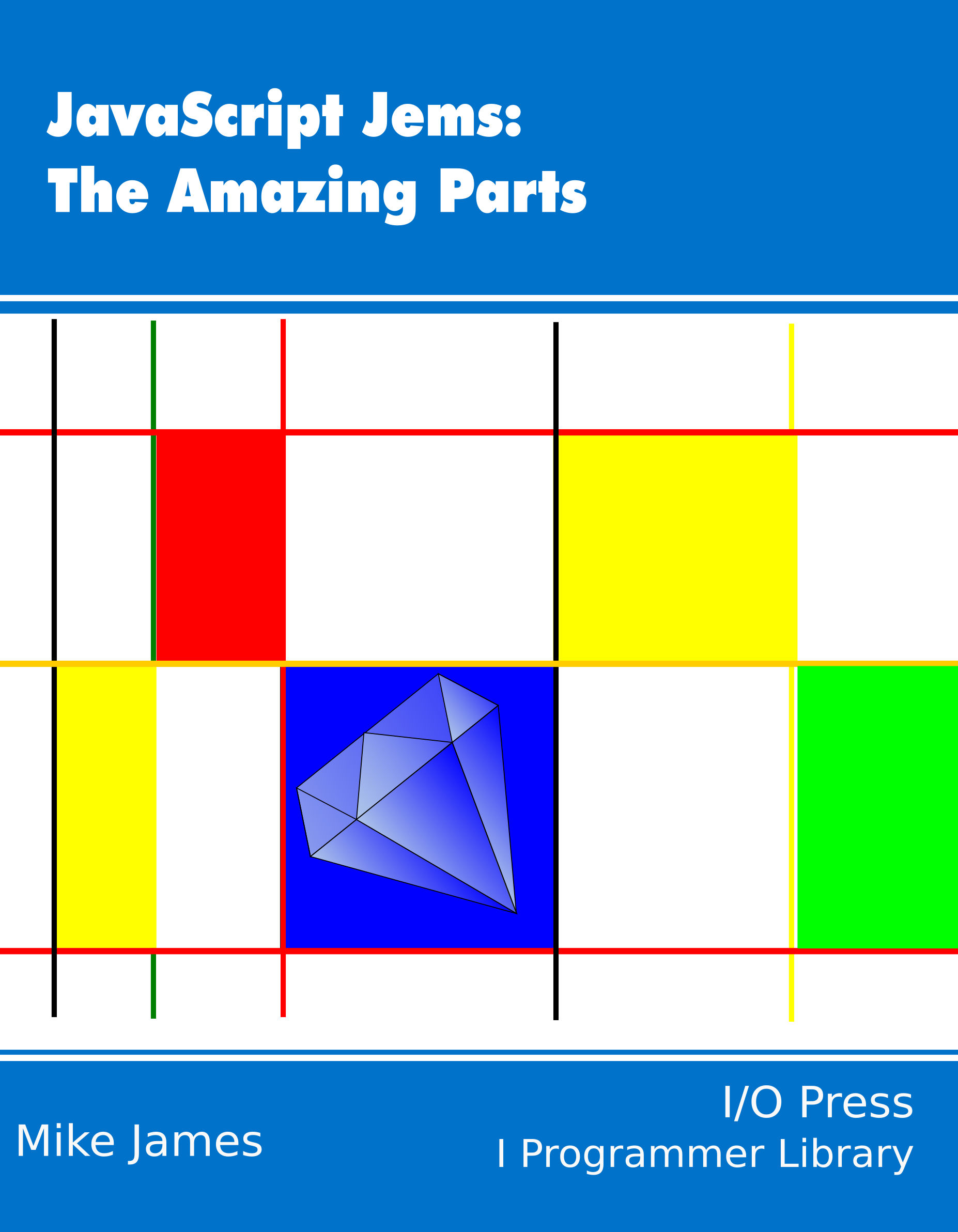
JavaScript Jems - Functional And Not Quite Functional
Original drafts of chapters.
Contents
- JavaScript Patterns
Why JavaScript is a Jem
- Objects with Values in JavaScript*
- Private Functions In JavaScript
- Chaining - Fluent Interfaces In JavaScript*
- Active Logic, Truthy and Falsey*
- The Confusing Comma In JavaScript*
- Self Modifying Code*
- Lambda expressions
- Meta Programming Using Proxy
- Master JavaScript Regular Expressions
- The Function Approach To Programming
The comma operator in JavaScript is really very simple and, to be 100% honest, you never need to use it. What is more you probably shouldn't use it - but is this a reason for not understanding it?
No, of course not.
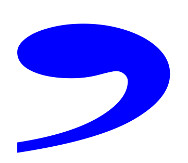
The Basic Comma
The comma operator fits in with a set of expression operators and it can be useful, but it has a tendency to be used in ways that confuse rather than clarify.
Part of the reason for this is that it is a comma, and commas have other meanings in JavaScript.
So to be 100% clear, the comma is only a comma operator when it acts on two expressions:
lefthand expression , righthand expression
Where expression is something that involves other operators, variables and functions.
What does the comma operator do?
It evaluates each of the expressions, starting with the lefthand expression and returns the value of the righthand expression.
For example
a = (1, 2);
stores 2 in a. Of course, the 1 and 2 are standing in for any complicated expression you care to think up, and you can use string expressions, functions and so on.
But why the parentheses?
The answer is that the comma operator has the lowest priority of all the JavaScript operators and without the parentheses the expression would be parsed as:
(a=1), 2;
which ends up storing 1 in a and throwing away the result of the righthand expression.
If you use more than one comma then, by the rules of operator precedence and left associativity, the result is that each expression is evaluated in turn from left to right and the last one is returned as the result.
That is:
(exp1, exp2, exp3, and so on expN)
evaluates exp1, exp2 etc. and returns expN as its result.
Side Effects
For simple comma expressions like:
a = (1, 2);
the value of the lefthand expression is always lost - so why bother?
The answer is that some operators have side effects and most functions have side effects.
For example, you can use:
a=(console.log(1),console.log(2),console.log(3),4);
and you will see 1,2,3 printed on the console and 4 stored in a.
Of course, given function calls and assignments are also statements we can use the semicolon:
console.log(1);console.log(2);console.log(3);a=4;
The point is that semicolons separate statements and commas separate expressions, and in some cases statements are also expressions. There are places where you can only use commas, but often you have a choice.
The problem here is that some programmers choose to use a comma just because it looks cool and this is just confusing.
|