Page 1 of 4 So you want to get up to speed with Python. Here's a lightening tour for the beginning to intermediate programmer who is already familiar with some fundamental programming ideas.
Programmer's Python Everything is an Object Second Edition
Is now available as a print book: Amazon
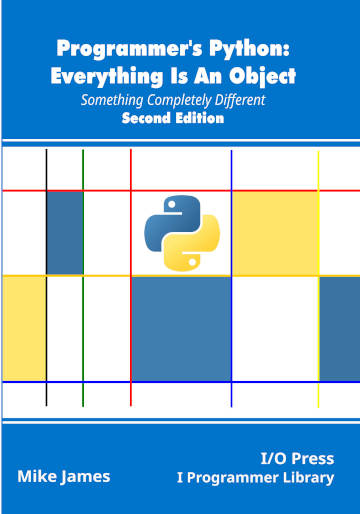
Contents
- Get Ready For The Python Difference
- Variables, Objects and Attributes
- The Function Object
- Scope, Lifetime and Closure
Extract 1: Local and Global
- Advanced Functions
- Decorators
- Class, Methods and Constructors
Extract 1: Objects Become Classes
- Inside Class ***NEW!
- Meeting Metaclasses
- Advanced Attributes
- Custom Attribute Access
- Single Inheritance
- Multiple Inheritance
- Class and Type
- Type Annotation
- Operator Overloading
- Python In Visual Studio Code
Extracts from the first edition
First the usual basics - skip if you already know about Python's birth and background:
And now for something completely different – Python
Well, if you are going to venture into Python you might as well get used to the Monty Python quotes, and expect references to spam (a type of meatloaf and an acquired taste), Brian and grail (references to two classic films).
Although it is true to say that Monty Python references seem to be getting thinner on the ground than they used to be. Perhaps it isn't as well known any more.Instead you tend to see illustrations and allusions to snakes, obviously pythons in particular. Originally there was nothing snaky about the language.
I will try to redress the balance slightly in this introduction.
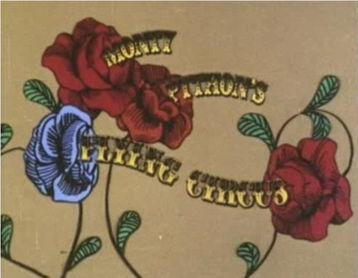
This is a brief introduction to Python for the beginning to intermediate programmer. It is assumed that you know enough about programming not to be confused by ideas such as variables, constants, loops and conditionals. It isn't a complete overview of Python more a guide to the things that an experienced programmer would notice or need to notice, in a first encounter.
Python is a free to use open source language. Developed by Guido van Rossum, an enthusiastic Monty Python fan, in 1990:

He is still very active on the project and is known affectionately in the Python community as Benevolent Dictator For Life or BDFL for short. Many of the characteristics of Python and the overall direction of the project are down to decisions made by Rossum.
Although it is copyrighted by van Rossum, you can use it and distribute it without charge, even for commercial use. You can download a Windows/Linux/Mac version complete with a visual development environment for free and the good news is that your Python application will often run irrespective of which platform you developed it on i.e. Python is largely platform independent.
The first question to answer is why bother?
Python may be a high quality language but so is Java!
Python is a phenomenon of modern programming. It is an interpreted object-oriented language that started out being simple and easy to use and has slowly collected a wide range of sophisticated features. The Python community is large and one of the most active. Python's excellent number crunching facilities have made it a number one choice for science and technology. In fact, in many cases data scientists prefer its general purpose approach to more directly data-oriented languages like R.
Having said all of this it also has to be said that Python has a slightly rough past which does hang around in the present. It wasn't particularly object oriented in its early days and today you can still write Python as if it was a scripting language. It is also dynamically typed. This is something that pleases many open minded programmers and infuriates the more strongly typed - you can guess which side of the argument I'm on.
In short, Python is easy to get started with, but it has a depth that you can make use of as you progress.
Getting a Python
If you want to try out Python then it couldn't be easier. Just go to www.python.org and download the version of Python you want to use.
This is our first problem.
Python comes in two distinct versions 2 and 3 and they aren't completely compatible. A decision was made to create a better version of Python even at the cost of making a non-compatible break with the current version. Python 3 was released on 3 December 2008 and at first it was difficult to know if you should use Python 2 or 3. Over time many of the incompatible elements in Python 3 were back ported to new version of Python 2 and the differences became less.
Now the choice of which Python to use is fairly obvious. If you don't have to work with legacy Python 2 code then learn Python 3. If you do have to work with legacy Python 2 code then learn Python 3 and convert the code to a only version of Python with a future.
Download the latest version of Python 3 and run the installer. Notice that if you are working with Linux then some distributions still use Python 2 as their default distribution - make sure to install the latest Python 3.
After installation is complete you can try Python out either by using the command line interpreter or the IDLE visual IDE which is installed automatically.
Eventually you most probably will want to move to a more complete IDE than IDLE and the only problem here is that there are lots to choose from. To get started however IDLE will do.
If you start IDLE you can try out your first Python program by simply typing in:
2+2
and you will see 4 displayed..
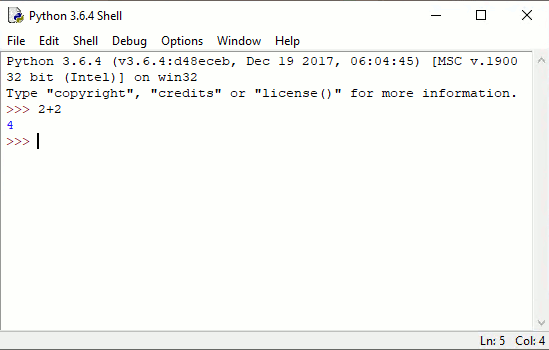
Python is an interpreted language and whatever you type in is obeyed at once. Python is dynamic and variables can be assigned to without any need to declare them first.
Built in variable types include integers, floating point, complex numbers, unlimited precision integers and strings.
Notice that already we are moving into some unusual areas – most languages don’t bother providing complex numbers or integers with as many digits as you like.
What is more, Python decides the type of variable needed according to what you try to store in it.
For example,
x=2+3j
is complex,
x=1
is integer and
x=123456578901234567891234567890123456789
is a long integer.
You also get all of the standard arithmetic operations and comparisons, plus some more interesting binary operations such as >> shift and xor but you can learn about these as you go along.
If you type any of these assignments into IDLE they will be executed. To see the result simply type the variable name and enter a return and IDLE will show you the contents of the variable.
Strings
The fundamental way of working with text in Python is to use a string.
Strings work much like they used to in Basic in that there is no equivalent to a fundamental character type – if you want to work with a single character just use a one-character string.
Strings support concatenation and slicing.
For example:
s = “Spam” + “Spam”
gives “SpamSpam” and
s[i:j]
is a slice of the string from offset i up to but not including offset j.The first character of a string is at offset 0 so
s[0,1]
gives S and
s[0,2]
gives Sa and so on.
Negative offsets are from the right hand end of the string.
There are so many ways of writing slices and string operators in general that it deserves a complete chapter.
You can also use a repeat operator
s="Spam" s=s*3
is three times the amount of Spam i.e. SpamSpamSpam.
You can also make use of formatting operations very similar to the C formatting codes. For example, %%d formats a decimal integer into a string.
At this stage suffice it to say that Python has sufficient string handling to make most tasks relatively easy compared to the tricks you have to perform when using C or Java. The ability to handle text easily is one of Python's strong points.
More on strings in another chapter.
|