Page 1 of 2 Python is one of those select languages that make functions first class object. Yet you can use it for years and never even notice that it is an object-oriented language. What makes functions as objects special?
Programmer's Python Everything is an Object Second Edition
Is now available as a print book: Amazon
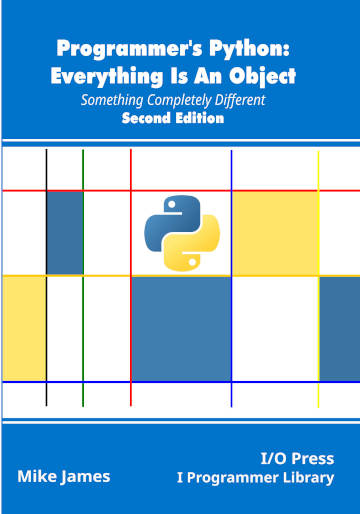
Contents
- Get Ready For The Python Difference
- Variables, Objects and Attributes
- The Function Object
- Scope, Lifetime and Closure
Extract 1: Local and Global
- Advanced Functions
- Decorators
- Class, Methods and Constructors
Extract 1: Objects Become Classes
- Inside Class ***NEW!
- Meeting Metaclasses
- Advanced Attributes
- Custom Attribute Access
- Single Inheritance
- Multiple Inheritance
- Class and Type
- Type Annotation
- Operator Overloading
- Python In Visual Studio Code
Extracts from the first edition
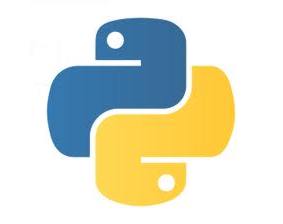
The Function Object
The Python function object is one of the reasons why programmers can completely miss the fact that Python is object-oriented. A Python function doesn’t give away the fact that it is an object as it can be used as if it was just a function, a function as you would find in almost any language. However, the Python function is an object and knowing this makes many things so much easier and so much more logical.
Before we learn about function objects let’s look at some of the more basic features of Python functions.
The Function Definition
A function is defined using def:
def sum(a,b):
c=a+b
return c
This looks like a function definition in many other languages and this is the intent.
You can call a function using the call operator () which is also used to specify arguments for the parameters.
For example:
print(sum(1,2))
prints the return value 3.
Notice that a function is not executed when it is defined, only when it is explicitly executed, but as we will discover something does happen during a function definition.
A function doesn’t need to have a return statement and doesn’t need to return a value. By default a function that doesn’t return a value returns the special value None which is generally discarded.
Variables that are defined within the function are added to the function’s local table which is different from its dictionary object. They are local variables in the sense that they have nothing to do with any variables with the same names elsewhere in the program. Unlike attributes, local variables only exist while the function is executing.
Pass-by-Object Reference
Parameters are added to the local table and hence behave like local variables. They are always passed by value but as the value of any variable in Python is a reference to an object this behaves more like pass by reference – sometimes called pass-by-object reference.
To make this clear we need more than one example.
First we need an object to use to pass to the function:
class MyClass:
a=0
The function simply attempts to change the value of its parameter:
def myFunction(x):
x=1
print(x)
Now when we call the function:
myFunction(MyClass)
print(MyClass.a)
what happens?
The answer is that the reference in MyClass is copied into the parameter x, then this is overwritten by a reference to an integer object. This means that we see 1 printed by the function. When the function returns, MyClass still references the original object and its a attribute is unchanged and still zero.
This means changes to parameters within functions have no effect on variables in the calling program.
However, this doesn’t mean that functions cannot change things in the calling program.
For example, consider:
def myFunction(x):
x.a = 1
print(x.a)
When this is called using:
myFunction(MyClass)
print(MyClass.a)
the same things occur – the reference in MyClass is copied into x, but then x.a which is the attribute on the same object that MyClass references is changed to 1. So the print in the function prints 1 but so does the print in the calling program.
Attributes of objects in the calling program can be changed by a function.
|