Page 1 of 4 There are moves to bring strong typing into Python and it isn’t a bad idea, but you need to understand type and its role in programming to make good use of it, or to decide if it is essential or optional.
Programmer's Python Everything is an Object Second Edition
Is now available as a print book: Amazon
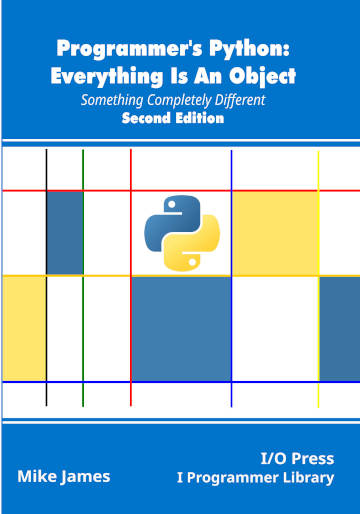
Contents
- Get Ready For The Python Difference
- Variables, Objects and Attributes
- The Function Object
- Scope, Lifetime and Closure
Extract 1: Local and Global
- Advanced Functions
- Decorators
- Class, Methods and Constructors
Extract 1: Objects Become Classes
- Inside Class ***NEW!
- Meeting Metaclasses
- Advanced Attributes
- Custom Attribute Access
- Single Inheritance
- Multiple Inheritance
- Class and Type
- Type Annotation
- Operator Overloading
- Python In Visual Studio Code
Extracts from the first edition
<ASIN:1871962749>
<ASIN:1871962595>
<ASIN:1871962765>
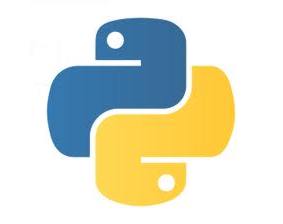
It is usually assumed that strong typing is essential to a programming language that is going to be used to create bug-free secure programs, but there is very little evidence for this point of view. Where a study has shown a slight advantage, no attempt has been made to find out what has been lost. Strong typing finds problems at compile time or in Python’s case edit time that could often be found just as easily by a static code analyzer – or indeed by running the program.
This said, there are some very clear and simple examples where indicating the type of a variable seems to be useful and natural – for example specifying the parameter types in a function. The problem is that when you try to describe the type of every variable or object in your program things very rapidly become very complicated with the need for type variables, generics and variance to describe them adequately. It may be that these complications make it possible to make errors that would not be errors without their introduction.
A cynical point of view is that strong typing finds many errors that would not be errors if you were not using it.
This said it does have its appeal at least at first so let’s find out what it is and how it works. And remember, currently its use is optional and it changes almost nothing about the way Python works.
Strong Typing
It once was the overwhelming freedom of Python that you could simply ignore type and it still is but the forces of strong typing are closing in with each new version.
Currently, typing in Python is optional and gradual and it is still evolving.
As remarked earlier, it is often said that Python’s default typing is strong and dynamic. This is supposed to mean that objects have a type and variables can change their type according to what is assigned to them.
But again as already commented, it is just as accurate to say that Python’s objects are dynamically typed as you can change their type at runtime and that its variables are un-typed because no use is made of any inferred type they might have.
This is powerful but there are times when you can’t help but feel the need to indicate what the parameters of a function are to be replaced with, i.e. what are acceptable arguments. This sort of pressure is pushing Python towards an optional system of type hints rather than the arguably better route of creating static analysis tools.
It might be too early to write a definitive account of type hints, but as with all things Python it isn’t just your average approach to type and it is worth knowing about, if only to find the direction that it is heading in.
In other languages type is tied to the idea of class, and class and type are almost inseparable. As you build a class hierarchy you are also building a type hierarchy. The principle of strong typing is that every variable has to have a declared type.
So in Java you might write:
int myVariable;
This declares that myVariable is of type int and this means it can only be used to reference an int or a subtype of int.
This makes it easy to detect mistakes before the program is run, but the mistakes that are picked up are usually easy to detect using other methods such as a static code checker. There is also an argument that typing variables adds to the range of ways you can make a mistake and these are the mistakes most often picked up by static typing.
For example:
int myVariable;
myVariable=”123”;
This would be a compile or edit time type error, but it is only an error because myVariable has been declared as of type int.
What strong typing does for you is that it states clearly what attributes the object is expected to have, and hence what operations you can apply to it.
You can reinterpret the earlier type error as – myVariable has been declared as an int and this means you can do arithmetic on it. Assigning a string to it therefore is a problem because you can’t do arithmetic on a string.
With this in mind we can now look at the way Python defines type separately from the idea of class in an effort to bring this sort of type checking to the language. The idea is to find a way to assign type to Python variables.
|