Page 1 of 3 Dictionaries are the key data structure in Python and you cannot avoid them. Find out how it all works in this extract from Programmer's Python: Everything is Data.
Programmer's Python Everything is Data
Is now available as a print book: Amazon
Contents
- Python – A Lightning Tour
- The Basic Data Type – Numbers
Extract: Bignum
- Truthy & Falsey
- Dates & Times
- Sequences, Lists & Tuples
Extract Sequences
- Strings
Extract Unicode Strings
- Regular Expressions
- The Dictionary
Extract The Dictionary
- Iterables, Sets & Generators
Extract Iterables
- Comprehensions
Extract Comprehensions
- Data Structures & Collections
Extract Stacks, Queues and Deques ***NEW!!!
- Bits & Bit Manipulation
Extract Bits and BigNum
- Bytes
Extract Bytes And Strings Extract Byte Manipulation
- Binary Files
- Text Files
- Creating Custom Data Classes
Extract A Custom Data Class
- Python and Native Code
Extract Native Code Appendix I Python in Visual Studio Code Appendix II C Programming Using Visual Studio Code
<ASIN:1871962765>
<ASIN:1871962749>
<ASIN:1871962595>
<ASIN:B0CK71TQ17>
<ASIN:187196265X>
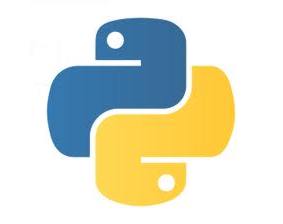
The sequence types are useful, but sometimes they don’t quite do what you want to. You can think of a sequence as associating integers to values:
0 → value, 1 → value
and so on.
Sometimes what you really need is a way to store a value in association with a more general data type than an integer. That is, you need to store values in association with specific key data:
key1 → value, key2 → value
and so on.
where key1 and key2 are almost any object. To do this we need to use a mapping type and the only standard mapping type that Python currently supports is the dictionary.
The Python dictionary is also known as an associative array or hash table. None of the names are particularly accurate or informative and they tend to color the way people think of the data structure. For example, in this case the dictionary isn't anything to do with putting things into alphabetic order. So let's try and forget what we call it and concentrate on what it does.
Basic use
A dictionary is a “machine” that you can use to store things in such a way that you can always find them again and find them very quickly. For example, suppose you want to keep track of how old people are. You can do this using a dictionary with almost no additional code.
For example to store a person's age you would use:
age = {}
age["Lucy"] = 19
The first instruction sets age to be an empty dictionary – it’s the curly brackets that make it a dictionary, just as square brackets make a list. After you have created an empty dictionary you can then store something in it, which is what the second line does.
To get Lucy's age back all we have to do is:
print age["Lucy"]
The dictionary is a machine that, when you give it two pieces of data – a key and a value, it stores the value in association with the key. If later you give it the key, it gives you the value you stored earlier. Notice that we use indexing, as in the case of the list, using square brackets. The only difference is that for a list the key has to be integer and is usually called the index and for a dictionary it can be any hashable object and we generally call it the key.
The value can be any type of data that you care to work with, and this is one of the powerful features of a dictionary.
So to summarize:
dict[key] = value
dict[key]
You can also create new dictionaries using the dict constructor:
-
dict() Creates an empty dictionary
-
dict(d) Creates a copy of the dictionary d
-
dict(iterable) Creates a new dictionary from the iterable
Each element of the iterable used to create a dictionary must be an iterable with two elements – the key and the value. A list, a tuple and a dictionary are all examples of iterables. which are covered in the next chapter.
The most commonly used is the final form of the constructor with the iterable being a list of (key.value) tuples. For example:
d=dict([("lucy",19),("harry",20),("ian",25),("sue",28)])
creates a dictionary with names as keys and ages as values. Notice that the list of tuples could just as easily be a tuple of tuples or a tuple of lists. The dict constructor using an iterable is very flexible.
|