Page 1 of 3 With its first class functions, JavaScript doesn't really need lambdas, but it has them and they are useful. Find out the mysteries of the lambda in Jem 11.
Now available as a book from your local Amazon.
JavaScript Jems: The Amazing Parts
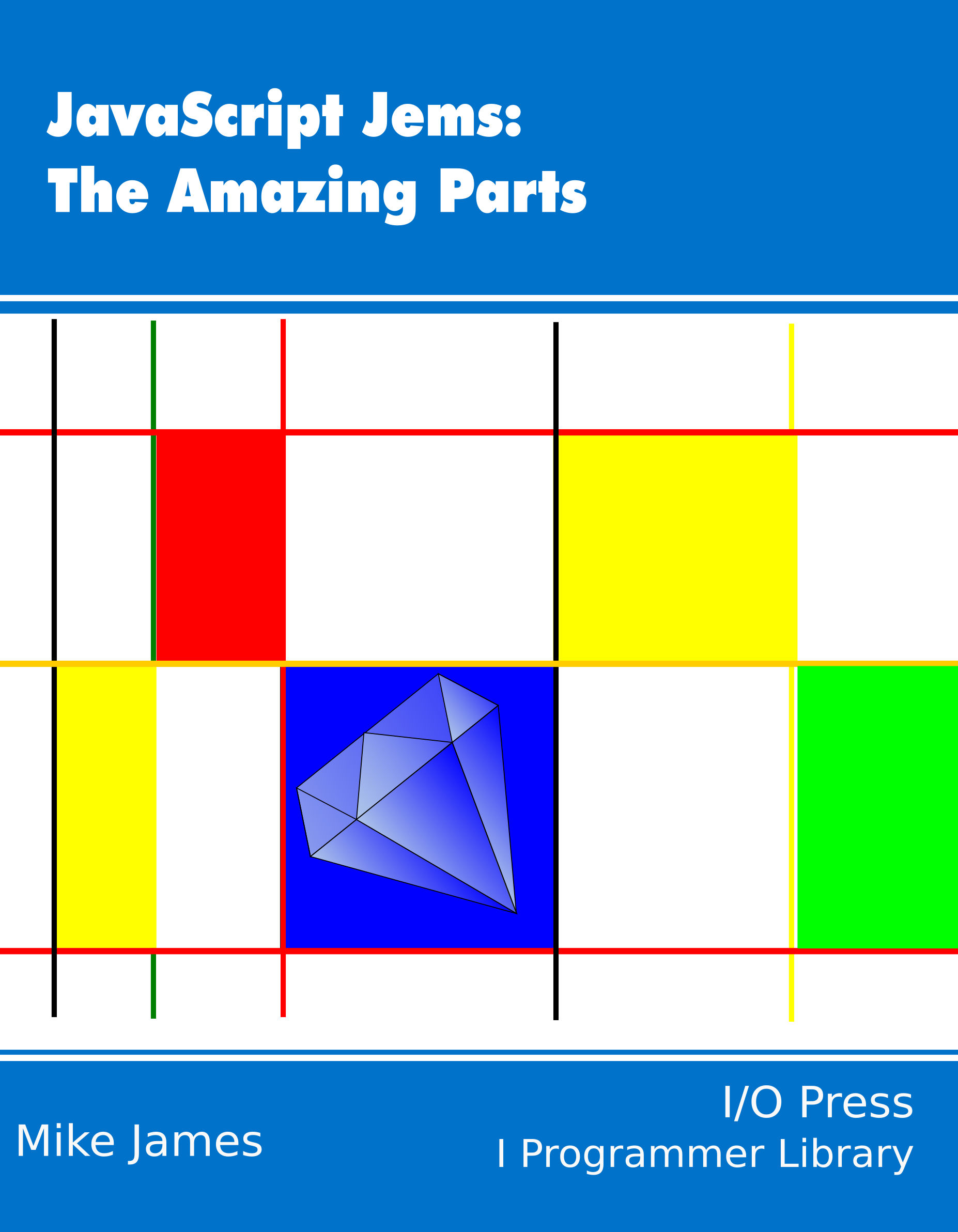
Contents
<ASIN:1871962579>
<ASIN:1871962560>
<ASIN:1871962501>
<ASIN:1871962528>
Jem 11
Lambda Expressions
“There may, indeed, be other applications of the system than its use as a logic.”
Alonzo Church
Lambda expressions sound advanced and impressive and just about every language has them or is adding them. Put simply, lambda expressions are something of a buzz word, so much that it's difficult to know what they really are all about.
One place you won’t hear much about lambda expressions is in JavaScript. You will even hear it said that JavaScript doesn't do lambdas, but this isn't the whole truth. JavaScript could always do lambda expressions, even before ES2015 introduced something equivalent to them.
First what is a lambda expression? Lambda expressions were invented by Alonzo Church and Stephen Kleene, two great computer scientists, back in the 1930s. In practice, this is fairly irrelevant in the sense that when most programmers use the term lambda expression they mean a function of a number of parameters that returns a single result and can be passed to other functions. The parameters and single result clearly aren’t a problem but what about passing a function to other functions?
Functions As Objects
We have already discovered, see Jem 3, that functions are objects that have some code associated with them that you can execute using the invocation () operator. What this means in practice is that a variable can store data or code with the only real difference being that the code can be executed.
This is often summarized by saying that functions in JavaScript are "first class" objects. That is, without the help of lambda expressions you can already pass functions to other functions and use them as if they were objects.
Contrast this to what happens in many other languages where functions are something completely different from objects and are usually thought of as being constituent parts of an object, namely methods. For example, consider the original version of Java, which as you will recall, has little to do with JavaScript other than its name. In Java everything is an object, but its objects have properties that can either be data or a functions. In this way functions are treated as methods and they always belong to an object. There is no sense in which a function can exist outside of an object. In Java, if you want to pass a function to a method then your only choice is to create an object which has that function as a method and pass the entire object. This is so unwieldy that recently Java introduced lambda expressions, which makes it possible to pass a function to a method without having to include it as a method in an object.
Any language that treats functions as something different from objects usually has to introduce lambda expressions or something similar. For example, C# introduced delegates, objects that wrap functions, and more recently full lambda expressions. All very complicated and not as elegant as JavaScript’s approach.
Put simply we need lambda expressions to provide us with functions that have a life of their own outside of objects and so that they can be passed around like objects.
JavaScript doesn’t need lambda expressions for either of these reasons as function are already objects and have a life of their own. You might say that this jem is about the fact that JavaScript doesn’t need to invent something to solve a problem it never had. Except, of course, ES2015 did just that and introduced something that looks like and behaves like a lambda function, see later.
Passing Functions
Given you can pass an object, i.e. as a parameter, into a function, it now seems entirely logical that you can pass a variable that just happens to reference a function object into another function. Recall that all parameters in JavaScript are passed by reference so just a pointer to the function is passed and this is efficient. If you don’t see why you would want to pass a function then you haven’t noticed that this is what you do every time you set up an event handler or a callback.
More generally, passing functions is also a fairly useful when you want to create a function that does a standard task, but with big variations. For example, the Array object has a method that will sort the array into order:
var list=new Array("A","AB","ABC","ABCD");
list.sort();
for(var i=0;i<list.length ;i++){
alert(list[i]);
}
What is perhaps slightly less well known is that the sort method can also take an optional function which it will use to compare the values of the array. This function is defined as:
function(a,b)
and it returns a negative value if a is smaller than b, zero if a is equal to b and a positive value if a is larger than b. For example:
compare=function(a,b){
return a.length-b.length;
}
used in:
list.sort(compare);
sorts the list of strings into order based on their length alone.
Other languages that don't have first class functions have to resort to delegate types, which are object wrappers for functions - or lambda expressions. So JavaScript’s functions almost do the same as a lambda but if you know about lambda functions you might object that having to first define the function and then use it in a call is less than elegant. Well, JavaScript has anonymous functions, which can be defined within the function call.
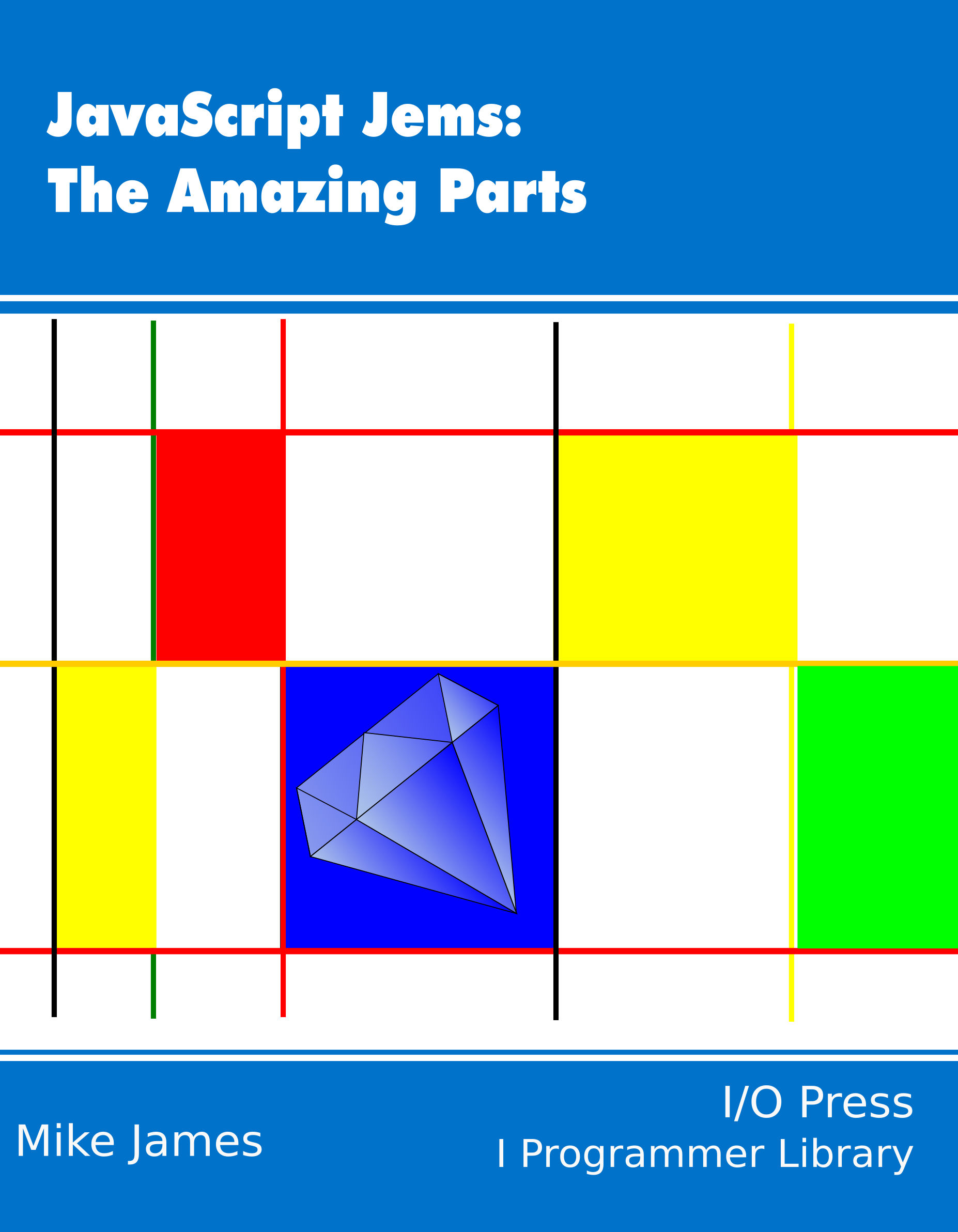
|