Page 1 of 3 Resources are a central part of Android programming, but how do you get at them from code and what are conditional resources? Here's how to do it in Kotlin, in an extract from my published book Android Programming in Kotlin: Starting With An App.
Android Programming In Kotlin Starting with an App
Covers Android Studio 3 and Constraint Layout.
Is now available as a print book:
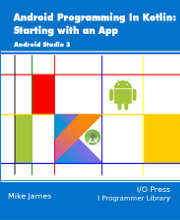
Buy from: Amazon
Contents
- Getting Started With Android Studio 3
- The Activity And The UI
Extract: Activity & UI
- Building The UI and a Calculator App
Extract: A First App
- Android Events
- Basic Controls
Extract Basic Controls Extract More Controls ***NEW!
- Layout Containers
Extract Layouts - LinearLayout
- The ConstraintLayout
Extract Bias & Chains
- Programming The UI
Extract Programming the UI Extract Layouts and Autonaming Components
- Menus & The Action Bar
- Menus, Context & Popup
- Resources
Extract Conditional Resources
- Beginning Bitmap Graphics
Extract Animation
- Staying Alive! Lifecycle & State
Extract State Managment
- Spinners
- Pickers
- ListView And Adapters
-
Android The Kotlin Way
If you are interested in creating custom template also see:
Custom Projects In Android Studio
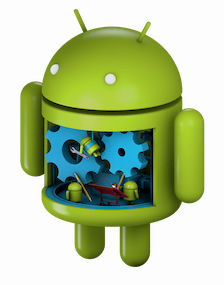
Accessing Resources in Code – The R Object
For much of the time you really don't need to bother with accessing resources in code because the job is done for you automatically. For example, if you assign a string resource to a button's text:
<Button
android:text="@string/Greeting"
then the system retrieves the string resource and sets the button's text property to it when the layout is inflated. You don't have to do anything to make it all work. However, sometimes resources are needed within the code of an app and you have to explicitly retrieve them.
When your app is compiled by Android Studio it automatically creates a resource id, a unique integer, for every resource in your res/ directory. These are stored in a generated class called R - for Resources. The directory structure starting with res/ is used to generate properties for the R object that allows you to find the id that corresponds to any resource. This means that a resource id is always named something like R.type.name. For example:
R.string.Greeting
retrieves the resource id for the string resource with resource name "Greeting" that is:
<string name="Greeting">Hello World</string>
Notice that the resource id is an integer and not the string it identifies.
So how do you convert a resource id to the resource value?
The first thing to say is that you don't always have to. There are many methods that accept a resource id as a parameter and will access the resource on your behalf. It is usually important to distinguish when a method is happy with a resource id and when you have to pass it the actual resource value.
If you do have to pass the resource, or you want to work with the resource, then you have to make use of the Resources object. This has a range of gettype(resource_id) methods that you can use to access any resource. For example, to get the string with the resource name "Greeting" you would write:
var myString=resources.getString(R.string.Greeting)
and myString would contain "Hello World". If you are not in the context of the activity you might have to use applicationContext.resources.
The only problem with using the Resources object is trying to work out which get methods you actually need.
There are also utility methods that will return any part or all of its resource name given the id:
Returns the entry name for a given resource identifier
Returns the full name for a given resource identifier
Returns the package name for a given resource identifier
Returns the type name for a given resource identifier.
There are also some methods that will process the resource as well as simply retrieve it. For example, if you have a raw XML resource, getXml(int id) returns an XmlResourceParser that lets you work through the XML retrieving tags, attributes, etc.
|